AraDisplay/AraCanvasMaker.cxx
00001 00002 00003 00004 00005 00006 00007 00008 #include <fstream> 00009 #include <iostream> 00010 #include "AraCanvasMaker.h" 00011 #include "AraGeomTool.h" 00012 #include "UsefulAtriStationEvent.h" 00013 #include "AraWaveformGraph.h" 00014 #include "AraEventCorrelator.h" 00015 #include "AraFFTGraph.h" 00016 #include "araSoft.h" 00017 00018 00019 #include "TString.h" 00020 #include "TObjArray.h" 00021 #include "TObjString.h" 00022 #include "TVector3.h" 00023 #include "TROOT.h" 00024 #include "TPaveText.h" 00025 #include "TPad.h" 00026 #include "TText.h" 00027 #include "TLatex.h" 00028 #include "TGraph.h" 00029 #include "TStyle.h" 00030 #include "TCanvas.h" 00031 #include "TAxis.h" 00032 #include "TH1.h" 00033 #include "TH2.h" 00034 #include "TList.h" 00035 #include "TFile.h" 00036 #include "TObject.h" 00037 #include "TTimeStamp.h" 00038 #include "TGeoManager.h" 00039 #include "TGeoVolume.h" 00040 #include "TView3D.h" 00041 00042 #include "FFTtools.h" 00043 00044 00045 AraCanvasMaker* AraCanvasMaker::fgInstance = 0; 00046 AraGeomTool *fACMGeomTool=0; 00047 00048 00049 00050 00051 AraWaveformGraph *grElec[CHANNELS_PER_ATRI]={0}; 00052 AraWaveformGraph *grElecFiltered[CHANNELS_PER_ATRI]={0}; 00053 AraWaveformGraph *grElecHilbert[CHANNELS_PER_ATRI]={0}; 00054 AraFFTGraph *grElecFFT[CHANNELS_PER_ATRI]={0}; 00055 AraFFTGraph *grElecAveragedFFT[CHANNELS_PER_ATRI]={0}; 00056 00057 AraWaveformGraph *grRFChan[CHANNELS_PER_ATRI]={0}; 00058 AraWaveformGraph *grRFChanFiltered[CHANNELS_PER_ATRI]={0}; 00059 AraWaveformGraph *grRFChanHilbert[CHANNELS_PER_ATRI]={0}; 00060 AraFFTGraph *grRFChanFFT[CHANNELS_PER_ATRI]={0}; 00061 AraFFTGraph *grRFChanAveragedFFT[CHANNELS_PER_ATRI]={0}; 00062 00063 00064 TH1D *AraCanvasMaker::getFFTHisto(int ant) 00065 { 00066 if(ant<0 || ant>=CHANNELS_PER_ATRI) return NULL; 00067 if(grRFChan[ant]) 00068 return grRFChan[ant]->getFFTHisto(); 00069 return NULL; 00070 00071 } 00072 00073 AraCanvasMaker::AraCanvasMaker(AraCalType::AraCalType_t calType) 00074 { 00075 //Default constructor 00076 fACMGeomTool=AraGeomTool::Instance(); 00077 fNumAntsInMap=4; 00078 fWebPlotterMode=0; 00079 fPassBandFilter=0; 00080 fNotchFilter=0; 00081 fLowPassEdge=200; 00082 fHighPassEdge=1200; 00083 fLowNotchEdge=235; 00084 fHighNotchEdge=500; 00085 fMinVoltLimit=-60; 00086 fMaxVoltLimit=60; 00087 fPhiMax=0; 00088 fMinClockVoltLimit=-200; 00089 fMaxClockVoltLimit=200; 00090 fAutoScale=1; 00091 fMinTimeLimit=0; 00092 fMaxTimeLimit=2000; 00093 fThisMinTime=0; 00094 fThisMaxTime=100; 00095 if(AraCalType::hasCableDelays(calType)) { 00096 fMinTimeLimit=-200; 00097 fMaxTimeLimit=150; 00098 } 00099 fMinPowerLimit=-60; 00100 fMaxPowerLimit=60; 00101 fMinFreqLimit=0; 00102 fMaxFreqLimit=1200; 00103 fWaveformOption=AraDisplayFormatOption::kWaveform; 00104 fRedoEventCanvas=0; 00105 //fRedoSurfCanvas=0; 00106 fLastWaveformFormat=AraDisplayFormatOption::kWaveform; 00107 fNewEvent=1; 00108 fCalType=calType; 00109 fCorType=AraCorrelatorType::kSphericalDist40; 00110 fgInstance=this; 00111 memset(grElec,0,sizeof(AraWaveformGraph*)*CHANNELS_PER_ATRI); 00112 memset(grElecFiltered,0,sizeof(AraWaveformGraph*)*CHANNELS_PER_ATRI); 00113 memset(grElecHilbert,0,sizeof(AraWaveformGraph*)*CHANNELS_PER_ATRI); 00114 memset(grElecFFT,0,sizeof(AraFFTGraph*)*CHANNELS_PER_ATRI); 00115 memset(grElecAveragedFFT,0,sizeof(AraFFTGraph*)*CHANNELS_PER_ATRI); 00116 00117 memset(grRFChan,0,sizeof(AraWaveformGraph*)*CHANNELS_PER_ATRI); 00118 memset(grRFChanFiltered,0,sizeof(AraWaveformGraph*)*CHANNELS_PER_ATRI); 00119 memset(grRFChanHilbert,0,sizeof(AraWaveformGraph*)*CHANNELS_PER_ATRI); 00120 memset(grRFChanFFT,0,sizeof(AraFFTGraph*)*CHANNELS_PER_ATRI); 00121 memset(grRFChanAveragedFFT,0,sizeof(AraFFTGraph*)*CHANNELS_PER_ATRI); 00122 switch(fCalType) { 00123 case AraCalType::kNoCalib: 00124 fMaxVoltLimit=3000; 00125 fMinVoltLimit=1000; 00126 break; 00127 case AraCalType::kJustUnwrap: 00128 fMinTimeLimit=0; 00129 fMaxTimeLimit=50; 00130 break; 00131 default: 00132 break; 00133 } 00134 00135 00136 } 00137 00138 AraCanvasMaker::~AraCanvasMaker() 00139 { 00140 //Default destructor 00141 } 00142 00143 00144 00145 //______________________________________________________________________________ 00146 AraCanvasMaker* AraCanvasMaker::Instance() 00147 { 00148 //static function 00149 return (fgInstance) ? (AraCanvasMaker*) fgInstance : new AraCanvasMaker(); 00150 } 00151 00152 00153 TPad *AraCanvasMaker::getEventInfoCanvas(UsefulAtriStationEvent *evPtr, TPad *useCan, Int_t runNumber) 00154 { 00155 static UInt_t lastEventNumber=0; 00156 static TPaveText *leftPave=0; 00157 static TPaveText *midLeftPave=0; 00158 static TPaveText *midRightPave=0; 00159 static TPaveText *rightPave=0; 00160 00161 00162 if(!fACMGeomTool) 00163 fACMGeomTool=AraGeomTool::Instance(); 00164 char textLabel[180]; 00165 TPad *topPad; 00166 if(!useCan) { 00167 topPad = new TPad("padEventInfo","padEventInfo",0.2,0.9,0.8,1); 00168 topPad->Draw(); 00169 } 00170 else { 00171 topPad=useCan; 00172 } 00173 if(1) { 00174 fNewEvent=1; 00175 topPad->Clear(); 00176 topPad->SetTopMargin(0.05); 00177 topPad->Divide(4,1); 00178 topPad->cd(1); 00179 if(leftPave) delete leftPave; 00180 leftPave = new TPaveText(0,0.1,1,0.9); 00181 leftPave->SetName("leftPave"); 00182 leftPave->SetBorderSize(0); 00183 leftPave->SetFillColor(0); 00184 leftPave->SetTextAlign(13); 00185 // if(runNumber) { 00186 sprintf(textLabel,"Run: %d",runNumber); 00187 TText *runText = leftPave->AddText(textLabel); 00188 runText->SetTextColor(50); 00189 // } 00190 sprintf(textLabel,"Event: %d",evPtr->eventNumber); 00191 TText *eventText = leftPave->AddText(textLabel); 00192 eventText->SetTextColor(50); 00193 sprintf(textLabel,"Event Id: %d",evPtr->eventId); 00194 TText *eventText2 = leftPave->AddText(textLabel); 00195 eventText2->SetTextColor(50); 00196 leftPave->Draw(); 00197 00198 00199 topPad->cd(2); 00200 gPad->SetRightMargin(0); 00201 gPad->SetLeftMargin(0); 00202 if(midLeftPave) delete midLeftPave; 00203 midLeftPave = new TPaveText(0,0.1,0.99,0.9); 00204 midLeftPave->SetName("midLeftPave"); 00205 midLeftPave->SetBorderSize(0); 00206 midLeftPave->SetTextAlign(13); 00207 TTimeStamp trigTime((time_t)evPtr->unixTime,(Int_t)1000*evPtr->unixTimeUs); 00208 sprintf(textLabel,"Time: %s",trigTime.AsString("s")); 00209 TText *timeText = midLeftPave->AddText(textLabel); 00210 timeText->SetTextColor(1); 00211 sprintf(textLabel,"Sub: %f",(trigTime.GetNanoSec()/1e9)); 00212 TText *timeText2 = midLeftPave->AddText(textLabel); 00213 timeText2->SetTextColor(1); 00214 midLeftPave->Draw(); 00215 // midLeftPave->Modified(); 00216 gPad->Modified(); 00217 gPad->Update(); 00218 00219 topPad->cd(3); 00220 if(midRightPave) delete midRightPave; 00221 midRightPave = new TPaveText(0,0.1,1,0.95); 00222 midRightPave->SetBorderSize(0); 00223 midRightPave->SetTextAlign(13); 00224 sprintf(textLabel,"PPS Num %d", 00225 evPtr->ppsNumber); 00226 midRightPave->AddText(textLabel); 00227 sprintf(textLabel,"Trig Time %d", 00228 evPtr->timeStamp); 00229 midRightPave->AddText(textLabel); 00230 // sprintf(textLabel,"Trig Type %d%d%d", 00231 // evPtr->isInTrigType(2), 00232 // evPtr->isInTrigType(1), 00233 // evPtr->isInTrigType(0)); 00234 // midRightPave->AddText(textLabel); 00235 // sprintf(textLabel,"Pattern %d%d%d%d%d%d%d%d", 00236 // evPtr->isInTrigPattern(7), 00237 // evPtr->isInTrigPattern(6), 00238 // evPtr->isInTrigPattern(5), 00239 // evPtr->isInTrigPattern(4), 00240 // evPtr->isInTrigPattern(3), 00241 // evPtr->isInTrigPattern(2), 00242 // evPtr->isInTrigPattern(1), 00243 // evPtr->isInTrigPattern(0)); 00244 midRightPave->Draw(); 00245 00246 00247 topPad->cd(4); 00248 if(rightPave) delete rightPave; 00249 rightPave = new TPaveText(0,0.1,1,0.95); 00250 rightPave->SetBorderSize(0); 00251 rightPave->SetTextAlign(13); 00252 sprintf(textLabel,"Num readout blocks %d", 00253 evPtr->numReadoutBlocks); 00254 rightPave->AddText(textLabel); 00255 sprintf(textLabel,"Blocks"); 00256 rightPave->AddText(textLabel); 00257 sprintf(textLabel,"%d -- ",0); 00258 for(int i=0;i<evPtr->numReadoutBlocks;i++) { 00259 if(i>0 && i%4==0) { 00260 rightPave->AddText(textLabel); 00261 sprintf(textLabel,"%d -- ",i); 00262 } 00263 sprintf(textLabel,"%s %d",textLabel,evPtr->blockVec[i].getBlock()); 00264 } 00265 rightPave->AddText(textLabel); 00266 00267 rightPave->Draw(); 00268 topPad->Update(); 00269 topPad->Modified(); 00270 00271 lastEventNumber=evPtr->eventNumber; 00272 } 00273 00274 return topPad; 00275 } 00276 00277 00278 TPad *AraCanvasMaker::quickGetEventViewerCanvasForWebPlottter(UsefulAtriStationEvent *evPtr, TPad *useCan) 00279 { 00280 TPad *retCan=0; 00281 fWebPlotterMode=1; 00282 // static Int_t lastEventView=0; 00283 int foundTimeRange = 0; 00284 00285 if(fAutoScale) { 00286 fMinVoltLimit=1e9; 00287 fMaxVoltLimit=-1e9; 00288 fMinClockVoltLimit=1e9; 00289 fMaxClockVoltLimit=-1e9; 00290 } 00291 00292 00293 00294 for(int chan=0;chan<CHANNELS_PER_ATRI;chan++) { 00295 if(grElec[chan]) delete grElec[chan]; 00296 if(grElecFFT[chan]) delete grElecFFT[chan]; 00297 if(grElecHilbert[chan]) delete grElecHilbert[chan]; 00298 grElec[chan]=0; 00299 grElecFFT[chan]=0; 00300 grElecHilbert[chan]=0; 00301 // if(grElecAveragedFFT[chan]) delete grElecAveragedFFT[chan]; 00302 00303 TGraph *grTemp = evPtr->getGraphFromElecChan(chan); 00304 if (!foundTimeRange && grTemp->GetN()) { 00305 foundTimeRange = 1; 00306 fThisMinTime=grTemp->GetX()[0]; 00307 fThisMaxTime=grTemp->GetX()[grTemp->GetN()-1]; 00308 } 00309 00310 // If GetN() returns 0, it's just an empty graph. 00311 // Draw will throw a bunch of stupid errors ("illegal number of points") 00312 // but other than that it'll be fine. Maybe we'll put a check somewhere 00313 // on Draw to shut up the errors. 00314 if (grTemp->GetN()) { 00315 if(grTemp->GetX()[0]<fThisMinTime) fThisMinTime=grTemp->GetX()[0]; 00316 if(grTemp->GetX()[grTemp->GetN()-1]>fThisMaxTime) fThisMaxTime=grTemp->GetX()[grTemp->GetN()-1]; 00317 } 00318 00319 grElec[chan] = new AraWaveformGraph(grTemp->GetN(),grTemp->GetX(),grTemp->GetY()); 00320 grElec[chan]->setElecChan(chan); 00321 // std::cout << evPtr->eventNumber << "\n"; 00322 // std::cout << surf << "\t" << chan << "\t" 00323 // << grElec[chan]->GetRMS(2) << std::endl; 00324 00325 if(fWaveformOption==AraDisplayFormatOption::kAveragedFFT) { 00326 TGraph *grTempFFT = grElec[chan]->getFFT(); 00327 grElecFFT[chan]=new AraFFTGraph(grTempFFT->GetN(), 00328 grTempFFT->GetX(), 00329 grTempFFT->GetY()); 00330 if(!grElecAveragedFFT[chan]) { 00331 grElecAveragedFFT[chan]=new AraFFTGraph(grTempFFT->GetN(), 00332 grTempFFT->GetX(), 00333 grTempFFT->GetY()); 00334 } 00335 else { 00336 grElecAveragedFFT[chan]->AddFFT(grElecFFT[chan]); 00337 } 00338 delete grTempFFT; 00339 } 00340 00341 00342 if(fAutoScale) { 00343 Int_t numPoints=grTemp->GetN(); 00344 Double_t *yVals=grTemp->GetY(); 00345 00346 for(int i=0;i<numPoints;i++) { 00347 if(yVals[i]<fMinVoltLimit) 00348 fMinVoltLimit=yVals[i]; 00349 if(yVals[i]>fMaxVoltLimit) 00350 fMaxVoltLimit=yVals[i]; 00351 } 00352 } 00353 00354 delete grTemp; 00355 } 00356 00357 foundTimeRange = 0; 00358 for(int rfchan=0;rfchan<CHANNELS_PER_ATRI;rfchan++) { 00359 if(grRFChan[rfchan]) delete grRFChan[rfchan]; 00360 if(grRFChanFFT[rfchan]) delete grRFChanFFT[rfchan]; 00361 if(grRFChanHilbert[rfchan]) delete grRFChanHilbert[rfchan]; 00362 grRFChan[rfchan]=0; 00363 grRFChanFFT[rfchan]=0; 00364 grRFChanHilbert[rfchan]=0; 00365 // if(grRFChanAveragedFFT[chan]) delete grRFChanAveragedFFT[chan]; 00366 //Need to work out how to do this 00367 TGraph *grTemp = evPtr->getGraphFromRFChan(rfchan); 00368 if (!foundTimeRange && grTemp->GetN()) { 00369 foundTimeRange = 1; 00370 fThisMinTime=grTemp->GetX()[0]; 00371 fThisMaxTime=grTemp->GetX()[grTemp->GetN()-1]; 00372 } 00373 00374 grRFChan[rfchan] = new AraWaveformGraph(grTemp->GetN(),grTemp->GetX(),grTemp->GetY()); 00375 grRFChan[rfchan]->setRFChan(rfchan, evPtr->stationId); 00376 // std::cout << evPtr->eventNumber << "\n"; 00377 // std::cout << surf << "\t" << chan << "\t" 00378 // << grElec[chan]->GetRMS(2) << std::endl; 00379 00380 00381 if(fWaveformOption==AraDisplayFormatOption::kAveragedFFT) { 00382 TGraph *grTempFFT = grRFChan[rfchan]->getFFT(); 00383 grRFChanFFT[rfchan]=new AraFFTGraph(grTempFFT->GetN(), 00384 grTempFFT->GetX(), 00385 grTempFFT->GetY()); 00386 if(!grRFChanAveragedFFT[rfchan]) { 00387 grRFChanAveragedFFT[rfchan]=new AraFFTGraph(grTempFFT->GetN(), 00388 grTempFFT->GetX(), 00389 grTempFFT->GetY()); 00390 } 00391 else { 00392 grRFChanAveragedFFT[rfchan]->AddFFT(grRFChanFFT[rfchan]); 00393 } 00394 delete grTempFFT; 00395 } 00396 00397 if(fAutoScale) { 00398 Int_t numPoints=grTemp->GetN(); 00399 Double_t *yVals=grTemp->GetY(); 00400 00401 for(int i=0;i<numPoints;i++) { 00402 if(yVals[i]<fMinVoltLimit) 00403 fMinVoltLimit=yVals[i]; 00404 if(yVals[i]>fMaxVoltLimit) 00405 fMaxVoltLimit=yVals[i]; 00406 } 00407 } 00408 delete grTemp; 00409 } 00410 00411 00412 if(fAutoScale) { 00413 if(fCalType!=AraCalType::kNoCalib && fCalType!=AraCalType::kJustUnwrap) { 00414 if(fMaxVoltLimit>-1*fMinVoltLimit) { 00415 fMinVoltLimit=-1*fMaxVoltLimit; 00416 } 00417 else { 00418 fMaxVoltLimit=-1*fMinVoltLimit; 00419 } 00420 } 00421 00422 00423 if(fMaxClockVoltLimit>-1*fMinClockVoltLimit) { 00424 fMinClockVoltLimit=-1*fMaxClockVoltLimit; 00425 } 00426 else { 00427 fMaxClockVoltLimit=-1*fMinClockVoltLimit; 00428 } 00429 } 00430 00431 00432 fRedoEventCanvas=0; 00433 00434 retCan=AraCanvasMaker::getCanvasForWebPlotter(evPtr,useCan); 00435 00436 00437 00438 return retCan; 00439 00440 } 00441 00442 TPad *AraCanvasMaker::getEventViewerCanvas(UsefulAtriStationEvent *evPtr, 00443 TPad *useCan) 00444 { 00445 TPad *retCan=0; 00446 00447 static UInt_t lastEventNumber=0; 00448 00449 int foundTimeRange = 0; 00450 00451 if(fAutoScale) { 00452 fMinVoltLimit=1e9; 00453 fMaxVoltLimit=-1e9; 00454 fMinClockVoltLimit=0; 00455 fMaxClockVoltLimit=0; 00456 } 00457 00458 00459 00460 for(int chan=0;chan<CHANNELS_PER_ATRI;chan++) { 00461 if(grElec[chan]) delete grElec[chan]; 00462 if(grElecFFT[chan]) delete grElecFFT[chan]; 00463 if(grElecHilbert[chan]) delete grElecHilbert[chan]; 00464 grElec[chan]=0; 00465 grElecFFT[chan]=0; 00466 grElecHilbert[chan]=0; 00467 00468 TGraph *grTemp = evPtr->getGraphFromElecChan(chan); 00469 if (grTemp->GetN() && !foundTimeRange) { 00470 fThisMinTime = grTemp->GetX()[0]; 00471 fThisMaxTime = grTemp->GetX()[grTemp->GetN()-1]; 00472 foundTimeRange = 1; 00473 } 00474 grElec[chan] = new AraWaveformGraph(grTemp->GetN(), grTemp->GetX(),grTemp->GetY()); 00475 grElec[chan]->setElecChan(chan); 00476 if(fWaveformOption==AraDisplayFormatOption::kAveragedFFT) { 00477 TGraph *grTempFFT = grElec[chan]->getFFT(); 00478 grElecFFT[chan]=new AraFFTGraph(grTempFFT->GetN(), 00479 grTempFFT->GetX(), 00480 grTempFFT->GetY()); 00481 if(!grElecAveragedFFT[chan]) { 00482 grElecAveragedFFT[chan]=new AraFFTGraph(grTempFFT->GetN(), 00483 grTempFFT->GetX(), 00484 grTempFFT->GetY()); 00485 } 00486 else { 00487 grElecAveragedFFT[chan]->AddFFT(grElecFFT[chan]); 00488 } 00489 delete grTempFFT; 00490 } 00491 00492 00493 if(fAutoScale) { 00494 Int_t numPoints=grTemp->GetN(); 00495 Double_t *yVals=grTemp->GetY(); 00496 00497 if(chan%8==7) { 00498 //Clock channel 00499 for(int i=0;i<numPoints;i++) { 00500 if(yVals[i]<fMinClockVoltLimit) 00501 fMinClockVoltLimit=yVals[i]; 00502 if(yVals[i]>fMaxClockVoltLimit) 00503 fMaxClockVoltLimit=yVals[i]; 00504 } 00505 } 00506 else{ 00507 for(int i=0;i<numPoints;i++) { 00508 if(yVals[i]<fMinVoltLimit) 00509 fMinVoltLimit=yVals[i]; 00510 if(yVals[i]>fMaxVoltLimit) 00511 fMaxVoltLimit=yVals[i]; 00512 } 00513 } 00514 } 00515 00516 delete grTemp; 00517 } 00518 // std::cout << "Limits\t" << fMinVoltLimit << "\t" << fMaxVoltLimit << "\n"; 00519 foundTimeRange = 0; 00520 00521 for(int rfchan=0;rfchan<CHANNELS_PER_ATRI;rfchan++) { 00522 if(grRFChan[rfchan]) delete grRFChan[rfchan]; 00523 if(grRFChanFFT[rfchan]) delete grRFChanFFT[rfchan]; 00524 if(grRFChanHilbert[rfchan]) delete grRFChanHilbert[rfchan]; 00525 grRFChan[rfchan]=0; 00526 grRFChanFFT[rfchan]=0; 00527 grRFChanHilbert[rfchan]=0; 00528 //Need to work out how to do this 00529 TGraph *grTemp = evPtr->getGraphFromRFChan(rfchan); 00530 if (grTemp->GetN() && !foundTimeRange) { 00531 fThisMinTime = grTemp->GetX()[0]; 00532 fThisMaxTime = grTemp->GetX()[grTemp->GetN()-1]; 00533 foundTimeRange = 1; 00534 } 00535 00536 if (grTemp->GetN()) { 00537 if(grTemp->GetX()[0]<fThisMinTime) fThisMinTime=grTemp->GetX()[0]; 00538 if(grTemp->GetX()[grTemp->GetN()-1]>fThisMaxTime) fThisMaxTime=grTemp->GetX()[grTemp->GetN()-1]; 00539 } 00540 grRFChan[rfchan] = new AraWaveformGraph(grTemp->GetN(),grTemp->GetX(),grTemp->GetY()); 00541 grRFChan[rfchan]->setRFChan(rfchan, evPtr->stationId); 00542 // std::cout << evPtr->eventNumber << "\n"; 00543 // std::cout << surf << "\t" << chan << "\t" 00544 // << grElec[chan]->GetRMS(2) << std::endl; 00545 00546 if(fWaveformOption==AraDisplayFormatOption::kAveragedFFT) { 00547 TGraph *grTempFFT = grRFChan[rfchan]->getFFT(); 00548 grRFChanFFT[rfchan]=new AraFFTGraph(grTempFFT->GetN(), 00549 grTempFFT->GetX(), 00550 grTempFFT->GetY()); 00551 if(!grRFChanAveragedFFT[rfchan]) { 00552 grRFChanAveragedFFT[rfchan]=new AraFFTGraph(grTempFFT->GetN(), 00553 grTempFFT->GetX(), 00554 grTempFFT->GetY()); 00555 } 00556 else { 00557 grRFChanAveragedFFT[rfchan]->AddFFT(grRFChanFFT[rfchan]); 00558 } 00559 delete grTempFFT; 00560 } 00561 if(fAutoScale) { 00562 Int_t numPoints=grTemp->GetN(); 00563 Double_t *yVals=grTemp->GetY(); 00564 00565 for(int i=0;i<numPoints;i++) { 00566 if(yVals[i]<fMinVoltLimit) 00567 fMinVoltLimit=yVals[i]; 00568 if(yVals[i]>fMaxVoltLimit) 00569 fMaxVoltLimit=yVals[i]; 00570 } 00571 } 00572 delete grTemp; 00573 } 00574 00575 if(fAutoScale) { 00576 00577 if(fCalType!=AraCalType::kNoCalib && fCalType!=AraCalType::kJustUnwrap) { 00578 if(fMaxVoltLimit>-1*fMinVoltLimit) { 00579 fMinVoltLimit=-1*fMaxVoltLimit; 00580 } 00581 else { 00582 fMaxVoltLimit=-1*fMinVoltLimit; 00583 } 00584 if(fMaxClockVoltLimit>-1*fMinClockVoltLimit) { 00585 fMinClockVoltLimit=-1*fMaxClockVoltLimit; 00586 } 00587 else { 00588 fMaxClockVoltLimit=-1*fMinClockVoltLimit; 00589 } 00590 } 00591 } 00592 00593 // std::cout << "Limits\t" << fMinVoltLimit << "\t" << fMaxVoltLimit << "\n"; 00594 00595 00596 fRedoEventCanvas=0; 00597 fNewEvent=0; 00598 00599 fRedoEventCanvas=0; 00600 if(fLastWaveformFormat!=fWaveformOption) fRedoEventCanvas=1; 00601 00602 00603 if(fCanvasLayout==AraDisplayCanvasLayoutOption::kElectronicsView) { 00604 retCan=AraCanvasMaker::getElectronicsCanvas(evPtr,useCan); 00605 } 00606 else if(fCanvasLayout==AraDisplayCanvasLayoutOption::kRFChanView) { 00607 retCan=AraCanvasMaker::getRFChannelCanvas(evPtr,useCan); 00608 } 00609 else if(fCanvasLayout==AraDisplayCanvasLayoutOption::kAntennaView) { 00610 retCan=AraCanvasMaker::getAntennaCanvas(evPtr,useCan); 00611 } 00612 else if(fCanvasLayout==AraDisplayCanvasLayoutOption::kIntMapView) { 00613 retCan=AraCanvasMaker::getIntMapCanvas(evPtr,useCan); 00614 } 00615 00616 00617 fLastWaveformFormat=fWaveformOption; 00618 fLastCanvasView=fCanvasLayout; 00619 00620 return retCan; 00621 00622 } 00623 00624 00625 TPad *AraCanvasMaker::getElectronicsCanvas(UsefulAtriStationEvent *evPtr,TPad *useCan) 00626 { 00627 // gStyle->SetTitleH(0.1); 00628 gStyle->SetOptTitle(0); 00629 00630 if(!fACMGeomTool) 00631 fACMGeomTool=AraGeomTool::Instance(); 00632 char textLabel[180]; 00633 char padName[180]; 00634 TPad *canElec=0; 00635 TPad *plotPad=0; 00636 if(!useCan) { 00637 canElec = (TPad*) gROOT->FindObject("canElec"); 00638 if(!canElec) { 00639 canElec = new TCanvas("canElec","canElec",1000,600); 00640 } 00641 canElec->Clear(); 00642 canElec->SetTopMargin(0); 00643 TPaveText *leftPave = new TPaveText(0.05,0.92,0.95,0.98); 00644 leftPave->SetBorderSize(0); 00645 sprintf(textLabel," Event %d",evPtr->eventNumber); 00646 TText *eventText = leftPave->AddText(textLabel); 00647 eventText->SetTextColor(50); 00648 leftPave->Draw(); 00649 plotPad = new TPad("canElecMain","canElecMain",0,0,1,0.9); 00650 plotPad->Draw(); 00651 } 00652 else { 00653 plotPad=useCan; 00654 } 00655 plotPad->cd(); 00656 setupElecPadWithFrames(plotPad); 00657 00658 00659 00660 int count=0; 00661 00662 // A 00663 // 1 3 5 7 9 00664 // 2 4 6 8 00665 // B 00666 // 1 3 5 7 9 00667 // 2 4 6 8 00668 // C 00669 // 1 3 5 7 9 00670 // 2 4 6 8 00671 00672 00673 00674 for(int row=0;row<RFCHAN_PER_DDA;row++) { 00675 for(int column=0;column<DDA_PER_ATRI;column++) { 00676 plotPad->cd(); 00677 int channel=row; 00678 int dda=column; 00679 int chanIndex=row+column*RFCHAN_PER_DDA; 00680 //if(channel>8) continue; 00681 00682 sprintf(padName,"elecChanPad%d",row+column*RFCHAN_PER_DDA); 00683 // std::cout << chanIndex << "\t" << labChip << "\t" << channel << "\t" << padName << "\n"; 00684 TPad *paddy1 = (TPad*) plotPad->FindObject(padName); 00685 paddy1->SetEditable(kTRUE); 00686 deleteTGraphsFromElecPad(paddy1,chanIndex); 00687 00688 00689 00690 if(fWaveformOption==AraDisplayFormatOption::kPowerSpectralDensity){ 00691 if(!grElecFFT[chanIndex]) { 00692 TGraph *grTemp=grElec[chanIndex]->getFFT(); 00693 grElecFFT[chanIndex]=new AraFFTGraph(grTemp->GetN(),grTemp->GetX(),grTemp->GetY()); 00694 delete grTemp; 00695 } 00696 grElecFFT[chanIndex]->Draw("l"); 00697 } 00698 else if(fWaveformOption==AraDisplayFormatOption::kAveragedFFT){ 00699 grElecAveragedFFT[chanIndex]->Draw("l"); 00700 } 00701 else if(fWaveformOption==AraDisplayFormatOption::kHilbertEnvelope) { 00702 if(!grElecHilbert[chanIndex]) { 00703 TGraph *grTemp=grElec[chanIndex]->getHilbert(); 00704 grElecHilbert[chanIndex]=new AraWaveformGraph(grTemp->GetN(),grTemp->GetX(),grTemp->GetY()); 00705 delete grTemp; 00706 } 00707 grElecHilbert[chanIndex]->Draw("l"); 00708 } 00709 else if(fWaveformOption==AraDisplayFormatOption::kWaveform){ 00710 00711 grElec[chanIndex]->Draw("l"); 00712 00713 00714 TList *listy = gPad->GetListOfPrimitives(); 00715 for(int i=0;i<listy->GetSize();i++) { 00716 TObject *fred = listy->At(i); 00717 TH1F *tempHist = (TH1F*) fred; 00718 if(tempHist->InheritsFrom("TH1")) { 00719 tempHist->GetXaxis()->SetRangeUser(fThisMinTime,fThisMaxTime); 00720 // std::cout << fThisMinTime << "\t" << fThisMaxTime << "\n"; 00721 if(fAutoScale) { 00722 if(channel<7) { 00723 tempHist->GetYaxis()->SetRangeUser(fMinVoltLimit,fMaxVoltLimit); 00724 } 00725 else { 00726 tempHist->GetYaxis()->SetRangeUser(fMinClockVoltLimit,fMaxClockVoltLimit); 00727 } 00728 } 00729 } 00730 } 00731 } 00732 00733 00734 count++; 00735 paddy1->SetEditable(kFALSE); 00736 } 00737 } 00738 00739 if(!useCan) 00740 return canElec; 00741 else 00742 return plotPad; 00743 00744 00745 } 00746 00747 00748 TPad *AraCanvasMaker::getCanvasForWebPlotter(UsefulAtriStationEvent *evPtr, 00749 TPad *useCan) 00750 { 00751 // gStyle->SetTitleH(0.1); 00752 gStyle->SetOptTitle(0); 00753 00754 if(!fACMGeomTool) 00755 fACMGeomTool=AraGeomTool::Instance(); 00756 char textLabel[180]; 00757 char padName[180]; 00758 TPad *canRFChan=0; 00759 TPad *plotPad=0; 00760 if(!useCan) { 00761 canRFChan = (TPad*) gROOT->FindObject("canRFChan"); 00762 if(!canRFChan) { 00763 canRFChan = new TCanvas("canRFChan","canRFChan",1000,600); 00764 } 00765 canRFChan->Clear(); 00766 canRFChan->SetTopMargin(0); 00767 TPaveText *leftPave = new TPaveText(0.05,0.92,0.95,0.98); 00768 leftPave->SetBorderSize(0); 00769 sprintf(textLabel,"Event %d",evPtr->eventNumber); 00770 TText *eventText = leftPave->AddText(textLabel); 00771 eventText->SetTextColor(50); 00772 leftPave->Draw(); 00773 plotPad = new TPad("canRFChanMain","canRFChanMain",0,0,1,0.9); 00774 plotPad->Draw(); 00775 } 00776 else { 00777 plotPad=useCan; 00778 } 00779 plotPad->cd(); 00780 setupRFChanPadWithFrames(plotPad); 00781 00782 00783 00784 int count=0; 00785 00786 00787 // 1 2 3 4 00788 // 5 6 7 8 00789 // 9 10 11 12 00790 // 13 14 15 16 00791 00792 for(int column=0;column<4;column++) { 00793 for(int row=0;row<4;row++) { 00794 plotPad->cd(); 00795 int rfChan=column+4*row; 00796 00797 sprintf(padName,"rfChanPad%d",column+4*row); 00798 TPad *paddy1 = (TPad*) plotPad->FindObject(padName); 00799 paddy1->SetEditable(kTRUE); 00800 deleteTGraphsFromRFPad(paddy1,rfChan); 00801 paddy1->cd(); 00802 00803 grRFChan[rfChan]->Draw("l"); 00804 00805 if(fAutoScale) { 00806 TList *listy = gPad->GetListOfPrimitives(); 00807 for(int i=0;i<listy->GetSize();i++) { 00808 TObject *fred = listy->At(i); 00809 TH1F *tempHist = (TH1F*) fred; 00810 if(tempHist->InheritsFrom("TH1")) { 00811 tempHist->GetYaxis()->SetRangeUser(fMinVoltLimit,fMaxVoltLimit); 00812 00813 } 00814 } 00815 } 00816 00817 00818 count++; 00819 paddy1->SetEditable(kFALSE); 00820 } 00821 } 00822 00823 if(!useCan) 00824 return canRFChan; 00825 else 00826 return plotPad; 00827 00828 00829 } 00830 00831 TPad *AraCanvasMaker::getRFChannelCanvas(UsefulAtriStationEvent *evPtr, 00832 TPad *useCan) 00833 { 00834 // gStyle->SetTitleH(0.1); 00835 gStyle->SetOptTitle(0); 00836 00837 if(!fACMGeomTool) 00838 fACMGeomTool=AraGeomTool::Instance(); 00839 char textLabel[180]; 00840 char padName[180]; 00841 TPad *canRFChan=0; 00842 TPad *plotPad=0; 00843 if(!useCan) { 00844 canRFChan = (TPad*) gROOT->FindObject("canRFChan"); 00845 if(!canRFChan) { 00846 canRFChan = new TCanvas("canRFChan","canRFChan",1000,600); 00847 } 00848 canRFChan->Clear(); 00849 canRFChan->SetTopMargin(0); 00850 TPaveText *leftPave = new TPaveText(0.05,0.92,0.95,0.98); 00851 leftPave->SetBorderSize(0); 00852 sprintf(textLabel,"Event %d",evPtr->eventNumber); 00853 TText *eventText = leftPave->AddText(textLabel); 00854 eventText->SetTextColor(50); 00855 leftPave->Draw(); 00856 plotPad = new TPad("canRFChanMain","canRFChanMain",0,0,1,0.9); 00857 plotPad->Draw(); 00858 } 00859 else { 00860 plotPad=useCan; 00861 } 00862 plotPad->cd(); 00863 setupRFChanPadWithFrames(plotPad); 00864 00865 00866 00867 for(int column=0;column<4;column++) { 00868 for(int row=0;row<4;row++) { 00869 plotPad->cd(); 00870 int rfChan=column+4*row; 00871 00872 sprintf(padName,"rfChanPad%d",column+4*row); 00873 TPad *paddy1 = (TPad*) plotPad->FindObject(padName); 00874 paddy1->SetEditable(kTRUE); 00875 deleteTGraphsFromRFPad(paddy1,rfChan); 00876 paddy1->cd(); 00877 00878 if(fWaveformOption==AraDisplayFormatOption::kPowerSpectralDensity){ 00879 if(!grRFChanFFT[rfChan]) { 00880 TGraph *grTemp=grRFChan[rfChan]->getFFT(); 00881 grRFChanFFT[rfChan]=new AraFFTGraph(grTemp->GetN(),grTemp->GetX(),grTemp->GetY()); 00882 delete grTemp; 00883 } 00884 grRFChanFFT[rfChan]->Draw("l"); 00885 } 00886 else if(fWaveformOption==AraDisplayFormatOption::kAveragedFFT){ 00887 grRFChanAveragedFFT[rfChan]->Draw("l"); 00888 } 00889 else if(fWaveformOption==AraDisplayFormatOption::kHilbertEnvelope) { 00890 if(!grRFChanHilbert[rfChan]) { 00891 TGraph *grTemp=grRFChan[rfChan]->getHilbert(); 00892 grRFChanHilbert[rfChan]=new AraWaveformGraph(grTemp->GetN(),grTemp->GetX(),grTemp->GetY()); 00893 delete grTemp; 00894 } 00895 grRFChanHilbert[rfChan]->Draw("l"); 00896 } 00897 else if(fWaveformOption==AraDisplayFormatOption::kWaveform) { 00898 grRFChan[rfChan]->Draw("l"); 00899 00900 if(fAutoScale) { 00901 TList *listy = gPad->GetListOfPrimitives(); 00902 for(int i=0;i<listy->GetSize();i++) { 00903 TObject *fred = listy->At(i); 00904 TH1F *tempHist = (TH1F*) fred; 00905 if(tempHist->InheritsFrom("TH1")) { 00906 tempHist->GetYaxis()->SetRangeUser(fMinVoltLimit,fMaxVoltLimit); 00907 } 00908 } 00909 } 00910 } 00911 00912 paddy1->SetEditable(kFALSE); 00913 } 00914 } 00915 00916 00917 if(!useCan) 00918 return canRFChan; 00919 else 00920 return plotPad; 00921 00922 } 00923 00924 00925 TPad *AraCanvasMaker::getAntennaCanvas(UsefulAtriStationEvent *evPtr, 00926 TPad *useCan) 00927 { 00928 // gStyle->SetTitleH(0.1); 00929 gStyle->SetOptTitle(0); 00930 00931 if(!fACMGeomTool) 00932 fACMGeomTool=AraGeomTool::Instance(); 00933 char textLabel[180]; 00934 char padName[180]; 00935 TPad *canRFChan=0; 00936 TPad *plotPad=0; 00937 if(!useCan) { 00938 canRFChan = (TPad*) gROOT->FindObject("canRFChan"); 00939 if(!canRFChan) { 00940 canRFChan = new TCanvas("canRFChan","canRFChan",1000,600); 00941 } 00942 canRFChan->Clear(); 00943 canRFChan->SetTopMargin(0); 00944 TPaveText *leftPave = new TPaveText(0.05,0.92,0.95,0.98); 00945 leftPave->SetBorderSize(0); 00946 sprintf(textLabel,"Event %d",evPtr->eventNumber); 00947 TText *eventText = leftPave->AddText(textLabel); 00948 eventText->SetTextColor(50); 00949 leftPave->Draw(); 00950 plotPad = new TPad("canRFChanMain","canRFChanMain",0,0,1,0.9); 00951 plotPad->Draw(); 00952 } 00953 else { 00954 plotPad=useCan; 00955 } 00956 plotPad->cd(); 00957 setupAntPadWithFrames(plotPad); 00958 00959 // int rfChanMap[4][4]={ 00960 AraAntPol::AraAntPol_t polMap[4][4]={{AraAntPol::kVertical,AraAntPol::kVertical,AraAntPol::kVertical,AraAntPol::kVertical}, 00961 {AraAntPol::kVertical,AraAntPol::kVertical,AraAntPol::kSurface,AraAntPol::kSurface}, 00962 {AraAntPol::kHorizontal,AraAntPol::kHorizontal,AraAntPol::kHorizontal,AraAntPol::kHorizontal}, 00963 {AraAntPol::kHorizontal,AraAntPol::kHorizontal,AraAntPol::kHorizontal,AraAntPol::kHorizontal}}; 00964 int antPolNumMap[4][4]={{0,1,2,3},{4,5,0,1},{0,1,2,3},{4,5,6,7}}; 00965 00966 00967 00968 00969 for(int row=0;row<4;row++) { 00970 for(int column=0;column<4;column++) { 00971 plotPad->cd(); 00972 int rfChan=fACMGeomTool->getRFChanByPolAndAnt(polMap[row][column],antPolNumMap[row][column], evPtr->stationId); 00973 // std::cout << row << "\t" << column << "\t" << rfChan << "\n"; 00974 00975 sprintf(padName,"antPad%d_%d",column,row); 00976 TPad *paddy1 = (TPad*) plotPad->FindObject(padName); 00977 paddy1->SetEditable(kTRUE); 00978 deleteTGraphsFromRFPad(paddy1,rfChan); 00979 paddy1->cd(); 00980 00981 if(fWaveformOption==AraDisplayFormatOption::kPowerSpectralDensity){ 00982 if(!grRFChanFFT[rfChan]) { 00983 TGraph *grTemp=grRFChan[rfChan]->getFFT(); 00984 grRFChanFFT[rfChan]=new AraFFTGraph(grTemp->GetN(),grTemp->GetX(),grTemp->GetY()); 00985 delete grTemp; 00986 } 00987 grRFChanFFT[rfChan]->Draw("l"); 00988 } 00989 else if(fWaveformOption==AraDisplayFormatOption::kAveragedFFT){ 00990 grRFChanAveragedFFT[rfChan]->Draw("l"); 00991 } 00992 else if(fWaveformOption==AraDisplayFormatOption::kHilbertEnvelope) { 00993 if(!grRFChanHilbert[rfChan]) { 00994 TGraph *grTemp=grRFChan[rfChan]->getHilbert(); 00995 grRFChanHilbert[rfChan]=new AraWaveformGraph(grTemp->GetN(),grTemp->GetX(),grTemp->GetY()); 00996 delete grTemp; 00997 } 00998 grRFChanHilbert[rfChan]->Draw("l"); 00999 } 01000 else if(fWaveformOption==AraDisplayFormatOption::kWaveform) { 01001 grRFChan[rfChan]->Draw("l"); 01002 01003 if(fAutoScale) { 01004 TList *listy = gPad->GetListOfPrimitives(); 01005 for(int i=0;i<listy->GetSize();i++) { 01006 TObject *fred = listy->At(i); 01007 TH1F *tempHist = (TH1F*) fred; 01008 if(tempHist->InheritsFrom("TH1")) { 01009 tempHist->GetYaxis()->SetRangeUser(fMinVoltLimit,fMaxVoltLimit); 01010 } 01011 } 01012 } 01013 } 01014 01015 paddy1->SetEditable(kFALSE); 01016 } 01017 } 01018 01019 01020 if(!useCan) 01021 return canRFChan; 01022 else 01023 return plotPad; 01024 01025 } 01026 01027 01028 TPad *AraCanvasMaker::getIntMapCanvas(UsefulAtriStationEvent *evPtr, 01029 TPad *useCan) 01030 { 01031 static UInt_t lastEventNumber=0; 01032 static UInt_t lastUnixTime=0; 01033 static UInt_t lastUnixTimeUs=0; 01034 Int_t sameEvent=0; 01035 if(lastEventNumber==evPtr->eventNumber) { 01036 if(lastUnixTime==evPtr->unixTime) { 01037 if(lastUnixTimeUs==evPtr->unixTimeUs) { 01038 sameEvent=1; 01039 } 01040 } 01041 } 01042 lastEventNumber=evPtr->eventNumber; 01043 lastUnixTime=evPtr->unixTime; 01044 lastUnixTimeUs=evPtr->unixTimeUs; 01045 01046 01047 // gStyle->SetTitleH(0.1); 01048 gStyle->SetOptTitle(0); 01049 01050 if(!fACMGeomTool) 01051 fACMGeomTool=AraGeomTool::Instance(); 01052 char textLabel[180]; 01053 char padName[180]; 01054 TPad *canIntMap=0; 01055 TPad *plotPad=0; 01056 if(!useCan) { 01057 canIntMap = (TPad*) gROOT->FindObject("canIntMap"); 01058 if(!canIntMap) { 01059 canIntMap = new TCanvas("canIntMap","canIntMap",1000,600); 01060 } 01061 canIntMap->Clear(); 01062 canIntMap->SetTopMargin(0); 01063 TPaveText *leftPave = new TPaveText(0.05,0.92,0.95,0.98); 01064 leftPave->SetBorderSize(0); 01065 sprintf(textLabel,"Event %d",evPtr->eventNumber); 01066 TText *eventText = leftPave->AddText(textLabel); 01067 eventText->SetTextColor(50); 01068 leftPave->Draw(); 01069 plotPad = new TPad("canIntMapMain","canIntMapMain",0,0,1,0.9); 01070 plotPad->Draw(); 01071 } 01072 else { 01073 plotPad=useCan; 01074 } 01075 plotPad->cd(); 01076 plotPad->Clear(); 01077 AraEventCorrelator *araCorPtr = AraEventCorrelator::Instance(fNumAntsInMap, evPtr->stationId); 01078 01079 static TH2D* histMapH=0; 01080 static TH2D* histMapV=0; 01081 plotPad->Divide(1,2); 01082 plotPad->cd(1); 01083 01084 if(histMapV) { 01085 if(!sameEvent) { 01086 delete histMapV; 01087 histMapV=0; 01088 } 01089 } 01090 01091 01092 // if(!histMapV) 01093 histMapV =araCorPtr->getInterferometricMap(evPtr,AraAntPol::kVertical,fCorType); 01094 histMapV->SetName("histMapV"); 01095 histMapV->SetTitle("Vertical Polarisation"); 01096 histMapV->SetXTitle("Azimuth (Degrees)"); 01097 histMapV->SetYTitle("Elevation (Degrees)"); 01098 histMapV->SetStats(0); 01099 // histMapV->SetMaximum(1); 01100 // histMapV->SetMinimum(-1); 01101 histMapV->Draw("colz"); 01102 plotPad->cd(2); 01103 if(histMapH) { 01104 if(!sameEvent) { 01105 delete histMapH; 01106 histMapH=0; 01107 } 01108 } 01109 01110 // if(!histMapH) 01111 histMapH =araCorPtr->getInterferometricMap(evPtr,AraAntPol::kHorizontal,fCorType); 01112 histMapH->SetName("histMapH"); 01113 histMapH->SetTitle("Hertical Polarisation"); 01114 histMapH->SetXTitle("Azimuth (Degrees)"); 01115 histMapH->SetYTitle("Elevation (Degrees)"); 01116 histMapH->SetStats(0); 01117 // histMapH->SetMaximum(1); 01118 // histMapH->SetMinimum(-1); 01119 01120 01121 histMapH->Draw("colz"); 01122 01123 if(!useCan) 01124 return canIntMap; 01125 else 01126 return plotPad; 01127 01128 } 01129 01130 01131 01132 01133 01134 01135 01136 void AraCanvasMaker::setupElecPadWithFrames(TPad *plotPad) 01137 { 01138 char textLabel[180]; 01139 char padName[180]; 01140 plotPad->cd(); 01141 if(fLastCanvasView!=AraDisplayCanvasLayoutOption::kElectronicsView) { 01142 plotPad->Clear(); 01143 } 01144 if(fRedoEventCanvas){ 01145 plotPad->Clear(); 01146 } 01147 01148 fLastCanvasView=AraDisplayCanvasLayoutOption::kElectronicsView; 01149 01150 01151 int numRows=RFCHAN_PER_DDA; 01152 int numCols=DDA_PER_ATRI; 01153 01154 static int elecPadsDone=0; 01155 if(elecPadsDone && !fRedoEventCanvas) { 01156 int errors=0; 01157 for(int i=0;i<numRows*numCols;i++) { 01158 sprintf(padName,"elecChanPad%d",i); 01159 TPad *paddy = (TPad*) plotPad->FindObject(padName); 01160 if(!paddy) 01161 errors++; 01162 } 01163 if(!errors) 01164 return; 01165 } 01166 01167 elecPadsDone=1; 01168 01169 01170 Double_t left[DDA_PER_ATRI]={0.04,0.28,0.52,0.76}; 01171 Double_t right[DDA_PER_ATRI]={0.28,0.52,0.76,0.99}; 01172 Double_t top[RFCHAN_PER_DDA]={0.93,0.80,0.69,0.58,0.47,0.36,0.25,0.14}; 01173 Double_t bottom[RFCHAN_PER_DDA]={0.80,0.69,0.58,0.47,0.36,0.25,0.14,0.03}; 01174 01175 //Now add some labels around the plot 01176 TLatex texy; 01177 texy.SetTextSize(0.03); 01178 texy.SetTextAlign(12); 01179 for(int column=0;column<numCols;column++) { 01180 sprintf(textLabel,"DDA %d",column+1); 01181 if(column==numCols-1) 01182 texy.DrawTextNDC(right[column]-0.1,0.97,textLabel); 01183 else 01184 texy.DrawTextNDC(right[column]-0.09,0.97,textLabel); 01185 } 01186 texy.SetTextAlign(21); 01187 texy.SetTextAngle(90); 01188 // texy.DrawTextNDC(left[0]-0.01,bottom[0],"A"); 01189 // texy.DrawTextNDC(left[0]-0.01,bottom[2],"B"); 01190 // texy.DrawTextNDC(left[0]-0.01,bottom[4],"C"); 01191 01192 // A 01193 // 1 3 5 7 9 01194 // 2 4 6 8 01195 // B 01196 // 1 3 5 7 9 01197 // 2 4 6 8 01198 // C 01199 // 1 3 5 7 9 01200 // 2 4 6 8 01201 01202 int count=0; 01203 01204 01205 01206 01207 for(int row=0;row<numRows;row++) { 01208 for(int column=0;column<numCols;column++) { 01209 plotPad->cd(); 01210 // if(row%2==1 && column==4) continue; 01211 sprintf(padName,"elecChanPad%d",row+column*RFCHAN_PER_DDA); 01212 TPad *paddy1 = new TPad(padName,padName,left[column],bottom[row],right[column],top[row]); 01213 paddy1->SetTopMargin(0); 01214 paddy1->SetBottomMargin(0); 01215 paddy1->SetLeftMargin(0); 01216 paddy1->SetRightMargin(0); 01217 if(column==numCols-1) 01218 paddy1->SetRightMargin(0.01); 01219 if(column==0) 01220 paddy1->SetLeftMargin(0.1); 01221 if(row==numRows-1) 01222 paddy1->SetBottomMargin(0.1); 01223 paddy1->Draw(); 01224 paddy1->cd(); 01225 01226 TH1F *framey=0; 01227 01228 01229 // std::cout << "Limits\t" << fMinVoltLimit << "\t" << fMaxVoltLimit << "\n"; 01230 01231 01232 if(fWaveformOption==AraDisplayFormatOption::kWaveform || fWaveformOption==AraDisplayFormatOption::kHilbertEnvelope) 01233 //framey = (TH1F*) paddy1->DrawFrame(0,fMinVoltLimit,250,fMaxVoltLimit); 01234 framey = (TH1F*) paddy1->DrawFrame(fMinTimeLimit,fMinVoltLimit,fMaxTimeLimit,fMaxVoltLimit); 01235 else if(fWaveformOption==AraDisplayFormatOption::kFFT || fWaveformOption==AraDisplayFormatOption::kAveragedFFT) 01236 framey = (TH1F*) paddy1->DrawFrame(fMinFreqLimit,fMinPowerLimit,fMaxFreqLimit,fMaxPowerLimit); 01237 01238 framey->GetYaxis()->SetLabelSize(0.08); 01239 framey->GetYaxis()->SetTitleSize(0.1); 01240 framey->GetYaxis()->SetTitleOffset(0.5); 01241 01242 if(row==numRows-1) { 01243 framey->GetXaxis()->SetLabelSize(0.09); 01244 framey->GetXaxis()->SetTitleSize(0.09); 01245 framey->GetYaxis()->SetLabelSize(0.09); 01246 framey->GetYaxis()->SetTitleSize(0.09); 01247 } 01248 if(fWebPlotterMode && column!=0) { 01249 framey->GetYaxis()->SetLabelSize(0); 01250 framey->GetYaxis()->SetTitleSize(0); 01251 framey->GetYaxis()->SetTitleOffset(0); 01252 } 01253 count++; 01254 } 01255 } 01256 01257 } 01258 01259 01260 01261 void AraCanvasMaker::setupRFChanPadWithFrames(TPad *plotPad) 01262 { 01263 static int rfChanPadsDone=0; 01264 char textLabel[180]; 01265 char padName[180]; 01266 plotPad->cd(); 01267 if(fLastCanvasView!=AraDisplayCanvasLayoutOption::kRFChanView) { 01268 plotPad->Clear(); 01269 } 01270 01271 if(fRedoEventCanvas && rfChanPadsDone){ 01272 plotPad->Clear(); 01273 } 01274 01275 fLastCanvasView=AraDisplayCanvasLayoutOption::kRFChanView; 01276 01277 if(rfChanPadsDone && !fRedoEventCanvas) { 01278 int errors=0; 01279 for(int rfChan=0;rfChan<CHANNELS_PER_ATRI;rfChan++) { 01280 sprintf(padName,"rfChanPad%d",rfChan); 01281 TPad *paddy = (TPad*) plotPad->FindObject(padName); 01282 if(!paddy) 01283 errors++; 01284 } 01285 if(!errors) 01286 return; 01287 } 01288 01289 01290 rfChanPadsDone=1; 01291 01292 01293 Double_t left[4]={0.04,0.27,0.50,0.73}; 01294 Double_t right[4]={0.27,0.50,0.73,0.96}; 01295 Double_t top[4]={0.95,0.72,0.49,0.26}; 01296 Double_t bottom[4]={0.72,0.49,0.26,0.03}; 01297 01298 //Now add some labels around the plot 01299 TLatex texy; 01300 texy.SetTextSize(0.03); 01301 texy.SetTextAlign(12); 01302 for(int column=0;column<4;column++) { 01303 sprintf(textLabel,"%d/%d",1+column,5+column); 01304 if(column==3) 01305 texy.DrawTextNDC(right[column]-0.12,0.97,textLabel); 01306 else 01307 texy.DrawTextNDC(right[column]-0.12,0.97,textLabel); 01308 } 01309 texy.SetTextAlign(21); 01310 texy.SetTextAngle(90); 01311 texy.DrawTextNDC(left[0]-0.01,bottom[0]+0.1,"1-4"); 01312 texy.DrawTextNDC(left[0]-0.01,bottom[1]+0.1,"5-8"); 01313 texy.DrawTextNDC(left[0]-0.01,bottom[2]+0.1,"9-12"); 01314 texy.DrawTextNDC(left[0]-0.01,bottom[3]+0.1,"13-16"); 01315 01316 01317 int count=0; 01318 01319 // 1 2 3 4 01320 // 5 6 7 8 01321 // 9 10 11 12 01322 // 13 14 15 16 01323 01324 for(int column=0;column<4;column++) { 01325 for(int row=0;row<4;row++) { 01326 plotPad->cd(); 01327 // int rfChan=column+4*row; 01328 sprintf(padName,"rfChanPad%d",column+4*row); 01329 TPad *paddy1 = new TPad(padName,padName,left[column],bottom[row],right[column],top[row]); 01330 paddy1->SetTopMargin(0); 01331 paddy1->SetBottomMargin(0); 01332 paddy1->SetLeftMargin(0); 01333 paddy1->SetRightMargin(0); 01334 if(column==3) 01335 paddy1->SetRightMargin(0.01); 01336 if(column==0) 01337 paddy1->SetLeftMargin(0.1); 01338 if(row==3) 01339 paddy1->SetBottomMargin(0.1); 01340 paddy1->Draw(); 01341 paddy1->cd(); 01342 TH1F *framey=0; 01343 if(fWaveformOption==AraDisplayFormatOption::kFFT || fWaveformOption==AraDisplayFormatOption::kAveragedFFT){ 01344 framey = (TH1F*) paddy1->DrawFrame(fMinFreqLimit,fMinPowerLimit,fMaxFreqLimit,fMaxPowerLimit); 01345 } 01346 else if(fWaveformOption==AraDisplayFormatOption::kWaveform || 01347 fWaveformOption==AraDisplayFormatOption::kHilbertEnvelope) { 01348 if(row<3) { 01349 framey = (TH1F*) paddy1->DrawFrame(fMinTimeLimit,fMinVoltLimit,fMaxTimeLimit,fMaxVoltLimit); 01350 } 01351 else{ 01352 framey = (TH1F*) paddy1->DrawFrame(fMinTimeLimit,fMinClockVoltLimit,fMaxTimeLimit,fMaxClockVoltLimit); 01353 } 01354 } 01355 01356 framey->GetYaxis()->SetLabelSize(0.1); 01357 framey->GetYaxis()->SetTitleSize(0.1); 01358 framey->GetYaxis()->SetTitleOffset(0.5); 01359 if(row==3) { 01360 framey->GetXaxis()->SetLabelSize(0.09); 01361 framey->GetXaxis()->SetTitleSize(0.09); 01362 framey->GetYaxis()->SetLabelSize(0.09); 01363 framey->GetYaxis()->SetTitleSize(0.09); 01364 } 01365 if(fWebPlotterMode && column!=0) { 01366 framey->GetYaxis()->SetLabelSize(0); 01367 framey->GetYaxis()->SetTitleSize(0); 01368 framey->GetYaxis()->SetTitleOffset(0); 01369 } 01370 01371 count++; 01372 } 01373 } 01374 } 01375 01376 01377 01378 void AraCanvasMaker::setupAntPadWithFrames(TPad *plotPad) 01379 { 01380 static int antPadsDone=0; 01381 char textLabel[180]; 01382 char padName[180]; 01383 plotPad->cd(); 01384 if(fLastCanvasView!=AraDisplayCanvasLayoutOption::kAntennaView) { 01385 plotPad->Clear(); 01386 } 01387 01388 if(fRedoEventCanvas && antPadsDone){ 01389 plotPad->Clear(); 01390 } 01391 01392 fLastCanvasView=AraDisplayCanvasLayoutOption::kAntennaView; 01393 01394 if(antPadsDone && !fRedoEventCanvas) { 01395 int errors=0; 01396 for(int column=0;column<4;column++) { 01397 for(int row=0;row<4;row++) { 01398 sprintf(padName,"antPad%d_%d",column,row); 01399 TPad *paddy = (TPad*) plotPad->FindObject(padName); 01400 if(!paddy) 01401 errors++; 01402 } 01403 } 01404 if(!errors) 01405 return; 01406 } 01407 01408 01409 antPadsDone=1; 01410 01411 01412 Double_t left[4]={0.04,0.27,0.50,0.73}; 01413 Double_t right[4]={0.27,0.50,0.73,0.96}; 01414 Double_t top[4]={0.95,0.72,0.49,0.26}; 01415 Double_t bottom[4]={0.72,0.49,0.26,0.03}; 01416 01417 //Now add some labels around the plot 01418 TLatex texy; 01419 texy.SetTextSize(0.03); 01420 texy.SetTextAlign(12); 01421 for(int column=0;column<4;column++) { 01422 sprintf(textLabel,"%d/%d",1+column,5+column); 01423 if(column==3) 01424 texy.DrawTextNDC(right[column]-0.12,0.97,textLabel); 01425 else 01426 texy.DrawTextNDC(right[column]-0.12,0.97,textLabel); 01427 } 01428 texy.SetTextAlign(21); 01429 texy.SetTextAngle(90); 01430 texy.DrawTextNDC(left[0]-0.01,bottom[0]+0.1,"V"); 01431 texy.DrawTextNDC(left[0]-0.01,bottom[1]+0.1,"V/S"); 01432 texy.DrawTextNDC(left[0]-0.01,bottom[2]+0.1,"H"); 01433 texy.DrawTextNDC(left[0]-0.01,bottom[3]+0.1,"H"); 01434 01435 01436 int count=0; 01437 01438 01439 01440 01441 for(int column=0;column<4;column++) { 01442 for(int row=0;row<4;row++) { 01443 plotPad->cd(); 01444 sprintf(padName,"antPad%d_%d",column,row); 01445 01446 TPad *paddy1 = new TPad(padName,padName,left[column],bottom[row],right[column],top[row]); 01447 paddy1->SetTopMargin(0); 01448 paddy1->SetBottomMargin(0); 01449 paddy1->SetLeftMargin(0); 01450 paddy1->SetRightMargin(0); 01451 if(column==3) 01452 paddy1->SetRightMargin(0.01); 01453 if(column==0) 01454 paddy1->SetLeftMargin(0.1); 01455 if(row==3) 01456 paddy1->SetBottomMargin(0.1); 01457 paddy1->Draw(); 01458 paddy1->cd(); 01459 TH1F *framey=0; 01460 if(fWaveformOption==AraDisplayFormatOption::kFFT || fWaveformOption==AraDisplayFormatOption::kAveragedFFT){ 01461 framey = (TH1F*) paddy1->DrawFrame(fMinFreqLimit,fMinPowerLimit,fMaxFreqLimit,fMaxPowerLimit); 01462 } 01463 else if(fWaveformOption==AraDisplayFormatOption::kWaveform || 01464 fWaveformOption==AraDisplayFormatOption::kHilbertEnvelope) { 01465 if(row<3) { 01466 framey = (TH1F*) paddy1->DrawFrame(fMinTimeLimit,fMinVoltLimit,fMaxTimeLimit,fMaxVoltLimit); 01467 } 01468 else{ 01469 framey = (TH1F*) paddy1->DrawFrame(fMinTimeLimit,fMinClockVoltLimit,fMaxTimeLimit,fMaxClockVoltLimit); 01470 } 01471 } 01472 01473 framey->GetYaxis()->SetLabelSize(0.1); 01474 framey->GetYaxis()->SetTitleSize(0.1); 01475 framey->GetYaxis()->SetTitleOffset(0.5); 01476 if(row==3) { 01477 framey->GetXaxis()->SetLabelSize(0.09); 01478 framey->GetXaxis()->SetTitleSize(0.09); 01479 framey->GetYaxis()->SetLabelSize(0.09); 01480 framey->GetYaxis()->SetTitleSize(0.09); 01481 } 01482 if(fWebPlotterMode && column!=0) { 01483 framey->GetYaxis()->SetLabelSize(0); 01484 framey->GetYaxis()->SetTitleSize(0); 01485 framey->GetYaxis()->SetTitleOffset(0); 01486 } 01487 count++; 01488 } 01489 } 01490 } 01491 01492 01493 01494 void AraCanvasMaker::deleteTGraphsFromElecPad(TPad *paddy,int chan) 01495 { 01496 paddy->cd(); 01497 if(fLastWaveformFormat==AraDisplayFormatOption::kWaveform) paddy->GetListOfPrimitives()->Remove(grElec[chan]); 01498 else if(fLastWaveformFormat==AraDisplayFormatOption::kFFT) paddy->GetListOfPrimitives()->Remove(grElecFFT[chan]); 01499 else if(fLastWaveformFormat==AraDisplayFormatOption::kAveragedFFT) paddy->GetListOfPrimitives()->Remove(grElecAveragedFFT[chan]); 01500 else if(fLastWaveformFormat==AraDisplayFormatOption::kHilbertEnvelope) paddy->GetListOfPrimitives()->Remove(grElecHilbert[chan]); 01501 // paddy->Update(); 01502 } 01503 01504 01505 void AraCanvasMaker::deleteTGraphsFromRFPad(TPad *paddy,int rfchan) 01506 { 01507 paddy->cd(); 01508 if(fLastWaveformFormat==AraDisplayFormatOption::kWaveform) paddy->GetListOfPrimitives()->Remove(grRFChan[rfchan]); 01509 else if(fLastWaveformFormat==AraDisplayFormatOption::kFFT) paddy->GetListOfPrimitives()->Remove(grRFChanFFT[rfchan]); 01510 else if(fLastWaveformFormat==AraDisplayFormatOption::kAveragedFFT) paddy->GetListOfPrimitives()->Remove(grRFChanAveragedFFT[rfchan]); 01511 else if(fLastWaveformFormat==AraDisplayFormatOption::kHilbertEnvelope) paddy->GetListOfPrimitives()->Remove(grRFChanHilbert[rfchan]); 01512 // paddy->Update(); 01513 } 01514 01515 01516 void AraCanvasMaker::resetAverage() 01517 { 01518 for(int chan=0;chan<CHANNELS_PER_ATRI;chan++) { 01519 if(grElecAveragedFFT[chan]) { 01520 delete grElecAveragedFFT[chan]; 01521 grElecAveragedFFT[chan]=0; 01522 } 01523 } 01524 for(int rfchan=0;rfchan<CHANNELS_PER_ATRI;rfchan++) { 01525 if(grRFChanAveragedFFT[rfchan]) { 01526 delete grRFChanAveragedFFT[rfchan]; 01527 grRFChanAveragedFFT[rfchan]=0; 01528 } 01529 } 01530 } 01531 01532 01533
Generated on Wed Aug 8 16:20:07 2012 for ARA ROOT Test Bed Software by
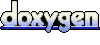