AraCorrelator/AraEventCorrelator.cxx
00001 #include <iostream> 00002 #include "AraEventCorrelator.h" 00003 #include "AraGeomTool.h" 00004 #include "AraAntennaInfo.h" 00005 #include "AraStationInfo.h" 00006 #include "UsefulIcrrStationEvent.h" 00007 #include "UsefulAtriStationEvent.h" 00008 #include "FFTtools.h" 00009 #include "TH2D.h" 00010 #include "TMath.h" 00011 00012 ClassImp(AraEventCorrelator); 00013 00014 AraEventCorrelator * AraEventCorrelator::fgInstance=0; 00015 00016 00017 AraEventCorrelator::AraEventCorrelator(Int_t numAnts, Int_t stationId) 00018 { 00019 //Default constructor 00020 fNumAnts=numAnts; 00021 fNumPairs=0; 00022 fStationId=stationId; 00023 for(int first=0;first<(fNumAnts-1);first++) { 00024 for(int second=first+1;second<fNumAnts;second++) { 00025 fFirstAnt[fNumPairs]=first; 00026 fSecondAnt[fNumPairs]=second; 00027 fNumPairs++; 00028 } 00029 } 00030 00031 00032 // fillDeltaTArrays(); 00033 fillAntennaPositions(); 00034 setupDeltaTInfinity(); 00035 setupDeltaT40m(); 00036 } 00037 00038 AraEventCorrelator::~AraEventCorrelator() 00039 { 00040 //Default destructor 00041 } 00042 00043 00044 //______________________________________________________________________________ 00045 AraEventCorrelator* AraEventCorrelator::Instance(Int_t numAnts, Int_t stationId) 00046 { 00047 //static function 00048 if(fgInstance) 00049 return fgInstance; 00050 00051 fgInstance = new AraEventCorrelator(numAnts, stationId); 00052 return fgInstance; 00053 } 00054 00055 void AraEventCorrelator::fillAntennaPositions() 00056 {AraGeomTool *araGeom=AraGeomTool::Instance(); 00057 for(int ant=0;ant<ANTS_PER_ICRR;ant++) { 00058 int antPolNum=araGeom->fStationInfo[fStationId].fAntInfo[ant].antPolNum; 00059 std::cerr << ant << "\t" << antPolNum << "\t" << araGeom->fStationInfo[fStationId].fAntInfo[ant].polType << "\n"; 00060 if(araGeom->fStationInfo[fStationId].fAntInfo[ant].polType==AraAntPol::kVertical) { 00061 if(antPolNum<7) { 00062 fRfChanVPol[antPolNum]=ant; 00063 fVPolPos[antPolNum][0]=araGeom->fStationInfo[fStationId].fAntInfo[ant].antLocation[0]; 00064 fVPolPos[antPolNum][1]=araGeom->fStationInfo[fStationId].fAntInfo[ant].antLocation[1]; 00065 fVPolPos[antPolNum][2]=araGeom->fStationInfo[fStationId].fAntInfo[ant].antLocation[2]; 00066 fVPolRho[antPolNum]=TMath::Sqrt(fVPolPos[antPolNum][0]*fVPolPos[antPolNum][0]+ 00067 fVPolPos[antPolNum][1]*fVPolPos[antPolNum][1]); 00068 fVPolPhi[antPolNum]=TMath::ATan2(fVPolPos[antPolNum][1],fVPolPos[antPolNum][0]); 00069 } 00070 } 00071 if(araGeom->fStationInfo[fStationId].fAntInfo[ant].polType==AraAntPol::kHorizontal) { 00072 if(antPolNum<7) { 00073 fRfChanHPol[antPolNum]=ant; 00074 fHPolPos[antPolNum][0]=araGeom->fStationInfo[fStationId].fAntInfo[ant].antLocation[0]; 00075 fHPolPos[antPolNum][1]=araGeom->fStationInfo[fStationId].fAntInfo[ant].antLocation[1]; 00076 fHPolPos[antPolNum][2]=araGeom->fStationInfo[fStationId].fAntInfo[ant].antLocation[2]; 00077 fHPolRho[antPolNum]=TMath::Sqrt(fHPolPos[antPolNum][0]*fHPolPos[antPolNum][0]+ 00078 fHPolPos[antPolNum][1]*fHPolPos[antPolNum][1]); 00079 fHPolPhi[antPolNum]=TMath::ATan2(fHPolPos[antPolNum][1],fHPolPos[antPolNum][0]); 00080 } 00081 } 00082 } 00083 for(int i=0;i<7;i++) { 00084 std::cout << "V\t" << i << "\t" << fVPolPos[i][0] << "\t" << fVPolPos[i][1] << "\t" << fVPolPos[i][2] << "\n"; 00085 } 00086 for(int i=0;i<7;i++) { 00087 std::cout << "H\t" << i << "\t" << fHPolPos[i][0] << "\t" << fHPolPos[i][1] << "\t" << fHPolPos[i][2] << "\n"; 00088 } 00089 00090 //Now fill the arrays with angles 00091 Double_t deltaPhi=360./NUM_PHI_BINS; 00092 Double_t deltaTheta=180./NUM_THETA_BINS; 00093 00094 for(int i=0;i<NUM_PHI_BINS;i++) { 00095 fPhiWaveDeg[i]=-180+0.5*deltaPhi+deltaPhi*i; 00096 fPhiWave[i]=fPhiWaveDeg[i]*TMath::DegToRad(); 00097 } 00098 for(int i=0;i<NUM_THETA_BINS;i++) { 00099 fThetaWaveDeg[i]=-90+0.5*deltaTheta+deltaTheta*i; 00100 fThetaWave[i]=fThetaWaveDeg[i]*TMath::DegToRad(); 00101 } 00102 } 00103 00104 00105 void AraEventCorrelator::setupDeltaTInfinity() 00106 { 00107 00108 00109 for(int pair=0;pair<fNumPairs;pair++) { 00110 int ind1=0; 00111 int ind2=0; 00112 getPairIndices(pair,ind1,ind2); 00113 for(int phiBin=0;phiBin<NUM_PHI_BINS;phiBin++) { 00114 for(int thetaBin=0;thetaBin<NUM_THETA_BINS;thetaBin++) { 00115 fVPolDeltaTInfinity[pair][phiBin][thetaBin]= 00116 calcDeltaTInfinity(fVPolPos[ind1],fVPolRho[ind1],fVPolPhi[ind1], 00117 fVPolPos[ind2],fVPolRho[ind2],fVPolPhi[ind2], 00118 fPhiWave[phiBin],fThetaWave[thetaBin]); 00119 fHPolDeltaTInfinity[pair][phiBin][thetaBin]= 00120 calcDeltaTInfinity(fHPolPos[ind1],fHPolRho[ind1],fHPolPhi[ind1], 00121 fHPolPos[ind2],fHPolRho[ind2],fHPolPhi[ind2], 00122 fPhiWave[phiBin],fThetaWave[thetaBin]); 00123 } 00124 } 00125 } 00126 00127 } 00128 00129 00130 void AraEventCorrelator::setupDeltaT40m() 00131 { 00132 Double_t R=41.8; 00133 00134 for(int pair=0;pair<fNumPairs;pair++) { 00135 int ind1=0; 00136 int ind2=0; 00137 getPairIndices(pair,ind1,ind2); 00138 // std::cout << "Starting pair " << pair << "\t" << ind1 << "\t" << ind2 << "\n"; 00139 for(int phiBin=0;phiBin<NUM_PHI_BINS;phiBin++) { 00140 for(int thetaBin=0;thetaBin<NUM_THETA_BINS;thetaBin++) { 00141 fVPolDeltaT40m[pair][phiBin][thetaBin]= 00142 calcDeltaTR(fVPolPos[ind1],fVPolRho[ind1],fVPolPhi[ind1], 00143 fVPolPos[ind2],fVPolRho[ind2],fVPolPhi[ind2], 00144 fPhiWave[phiBin],fThetaWave[thetaBin],R); 00145 fHPolDeltaT40m[pair][phiBin][thetaBin]= 00146 calcDeltaTR(fHPolPos[ind1],fHPolRho[ind1],fHPolPhi[ind1], 00147 fHPolPos[ind2],fHPolRho[ind2],fHPolPhi[ind2], 00148 fPhiWave[phiBin],fThetaWave[thetaBin],R); 00149 } 00150 } 00151 } 00152 00153 } 00154 00155 Double_t AraEventCorrelator::calcDeltaTInfinity(Double_t ant1[3],Double_t rho1, Double_t phi1, Double_t ant2[3],Double_t rho2, Double_t phi2, Double_t phiWave, Double_t thetaWave) 00156 { 00157 00158 Double_t d1=TMath::Cos(thetaWave)*(ant1[2]*TMath::Tan(thetaWave)+rho1*TMath::Cos(phi1-phiWave)); 00159 Double_t d2=TMath::Cos(thetaWave)*(ant2[2]*TMath::Tan(thetaWave)+rho2*TMath::Cos(phi2-phiWave)); 00160 Double_t t1t2=(d2-d1)*AraGeomTool::nTopOfIce/TMath::C(); 00161 t1t2*=1e9; 00162 return t1t2; 00163 // Double_t t2t1=(d1-d2)*AraGeomTool::nTopOfIce/TMath::C(); 00164 // t2t1*=1e9; 00165 // return t2t1; 00166 } 00167 00168 Double_t AraEventCorrelator::calcDeltaTR(Double_t ant1[3],Double_t rho1, Double_t phi1, Double_t ant2[3],Double_t rho2, Double_t phi2, Double_t phiWave, Double_t thetaWave, Double_t R) 00169 { 00170 00171 Double_t xs=R*TMath::Cos(thetaWave)*TMath::Cos(phiWave); 00172 Double_t ys=R*TMath::Cos(thetaWave)*TMath::Sin(phiWave); 00173 Double_t zs=R*TMath::Sin(thetaWave); 00174 00175 // if(phiWave*TMath::RadToDeg()>30 && phiWave*TMath::RadToDeg()<31) { 00176 // if(thetaWave*TMath::RadToDeg()>-45 && thetaWave*TMath::RadToDeg()<-44) { 00177 // std::cout << phiWave*TMath::RadToDeg() << "\t" << thetaWave*TMath::RadToDeg() << "\t" << R << "\t" << xs << "\t" << ys << "\t" << zs << "\n"; 00178 // } 00179 // } 00180 00181 Double_t d1=TMath::Sqrt((xs-ant1[0])*(xs-ant1[0])+(ys-ant1[1])*(ys-ant1[1])+(zs-ant1[2])*(zs-ant1[2])); 00182 Double_t d2=TMath::Sqrt((xs-ant2[0])*(xs-ant2[0])+(ys-ant2[1])*(ys-ant2[1])+(zs-ant2[2])*(zs-ant2[2])); 00183 00184 00185 // if(phiWave*TMath::RadToDeg()>30 && phiWave*TMath::RadToDeg()<31) { 00186 // if(thetaWave*TMath::RadToDeg()>-45 && thetaWave*TMath::RadToDeg()<-44) { 00187 // std::cout << d1 << "\t" << d2 << "\t" << d1-d2 << "\n"; 00188 // } 00189 // } 00190 00191 00192 Double_t t1t2=(d1-d2)*AraGeomTool::nTopOfIce/TMath::C(); 00193 t1t2*=1e9; 00194 return t1t2; 00195 } 00196 00197 void AraEventCorrelator::fillDeltaTArrays(AraCorrelatorType::AraCorrelatorType_t corType) { 00198 static AraCorrelatorType::AraCorrelatorType_t lastCorType=AraCorrelatorType::kNotACorType; 00199 if(lastCorType==corType) return; 00200 lastCorType=corType; 00201 00202 switch (corType) { 00203 case AraCorrelatorType::kSphericalDist40: 00204 for(int pair=0;pair<fNumPairs;pair++) { 00205 for(int phiBin=0;phiBin<NUM_PHI_BINS;phiBin++) { 00206 for(int thetaBin=0;thetaBin<NUM_THETA_BINS;thetaBin++) { 00207 fVPolDeltaT[pair][phiBin][thetaBin]=fVPolDeltaT40m[pair][phiBin][thetaBin]; 00208 fHPolDeltaT[pair][phiBin][thetaBin]=fHPolDeltaT40m[pair][phiBin][thetaBin]; 00209 } 00210 } 00211 } 00212 break; 00213 case AraCorrelatorType::kPlaneWave: 00214 default: 00215 for(int pair=0;pair<fNumPairs;pair++) { 00216 for(int phiBin=0;phiBin<NUM_PHI_BINS;phiBin++) { 00217 for(int thetaBin=0;thetaBin<NUM_THETA_BINS;thetaBin++) { 00218 fVPolDeltaT[pair][phiBin][thetaBin]=fVPolDeltaTInfinity[pair][phiBin][thetaBin]; 00219 fHPolDeltaT[pair][phiBin][thetaBin]=fHPolDeltaTInfinity[pair][phiBin][thetaBin]; 00220 } 00221 } 00222 } 00223 break; 00224 } 00225 } 00226 00227 00228 00229 void AraEventCorrelator::getPairIndices(int pair, int &ant1, int &ant2) 00230 { 00231 00232 if(pair>=0 && pair<fNumPairs) { 00233 ant1=fFirstAnt[pair]; 00234 ant2=fSecondAnt[pair]; 00235 } 00236 } 00237 00238 TH2D *AraEventCorrelator::getInterferometricMap(UsefulIcrrStationEvent *evPtr, AraAntPol::AraAntPol_t polType,AraCorrelatorType::AraCorrelatorType_t corType) 00239 { 00240 static int counter=0; 00241 fillDeltaTArrays(corType); 00242 //Now need to get correlations for the fNumPairs antenna pairs and then look up the correlation values of each one 00243 Double_t scale=1./fNumPairs; 00244 TH2D *histMap = new TH2D("histMap","histMap",NUM_PHI_BINS,-180,180,NUM_THETA_BINS,-90,90); 00245 TGraph *grRaw[fNumAnts]; 00246 TGraph *grInt[fNumAnts]; 00247 TGraph *grNorm[fNumAnts]; 00248 TGraph *grCor[fNumPairs]; 00249 for(int i=0;i<fNumAnts;i++) { 00250 grRaw[i]=0; 00251 grInt[i]=0; 00252 grNorm[i]=0; 00253 } 00254 for(int i=0;i<fNumPairs;i++) 00255 grCor[i]=0; 00256 00257 if(polType==AraAntPol::kVertical) { 00258 for(int ind=0;ind<fNumAnts;ind++) { 00259 // std::cerr << ind << "\t" << fRfChanVPol[ind] << "\n"; 00260 grRaw[ind]=evPtr->getGraphFromRFChan(fRfChanVPol[ind]); 00261 grInt[ind]=FFTtools::getInterpolatedGraph(grRaw[ind],0.5); 00262 grNorm[ind]=getNormalisedGraph(grInt[ind]); 00263 } 00264 // std::cerr << "Got graphs and made int maps\n"; 00265 00266 for(int pair=0;pair<fNumPairs;pair++) { 00267 int ind1=0; 00268 int ind2=0; 00269 getPairIndices(pair,ind1,ind2); 00270 // std::cerr << pair << "\t" << ind1 << "\t" << ind2 << "\n"; 00271 00272 grCor[pair]=FFTtools::getCorrelationGraph(grNorm[ind1],grNorm[ind2]); 00273 for(int phiBin=0;phiBin<NUM_PHI_BINS;phiBin++) { 00274 for(int thetaBin=0;thetaBin<NUM_THETA_BINS;thetaBin++) { 00275 //I think this is the correct equation to work out the bin number 00276 //Could just use TH2::GetBin(binx,biny) but the below should be faster 00277 Int_t globalBin=(phiBin+1)+(thetaBin+1)*(NUM_PHI_BINS+2); 00278 Double_t dt=fVPolDeltaT[pair][phiBin][thetaBin]; 00279 //Double_t corVal=grCor[pair]->Eval(dt); 00280 Double_t corVal=fastEvalForEvenSampling(grCor[pair],dt); 00281 corVal*=scale; 00282 Double_t binVal=histMap->GetBinContent(globalBin); 00283 histMap->SetBinContent(globalBin,binVal+corVal); 00284 } 00285 } 00286 } 00287 } 00288 else { 00289 for(int ind=0;ind<fNumAnts;ind++) { 00290 grRaw[ind]=evPtr->getGraphFromRFChan(fRfChanHPol[ind]); 00291 grInt[ind]=FFTtools::getInterpolatedGraph(grRaw[ind],0.5); 00292 grNorm[ind]=getNormalisedGraph(grInt[ind]); 00293 } 00294 00295 for(int pair=0;pair<fNumPairs;pair++) { 00296 int ind1=0; 00297 int ind2=0; 00298 getPairIndices(pair,ind1,ind2); 00299 grCor[pair]=FFTtools::getCorrelationGraph(grNorm[ind1],grNorm[ind2]); 00300 for(int phiBin=0;phiBin<NUM_PHI_BINS;phiBin++) { 00301 for(int thetaBin=0;thetaBin<NUM_THETA_BINS;thetaBin++) { 00302 //I think this is the correct equation to work out the bin number 00303 //Could just use TH2::GetBin(binx,biny) but the below should be faster 00304 Int_t globalBin=(phiBin+1)+(thetaBin+1)*(NUM_PHI_BINS+2); 00305 Double_t dt=fHPolDeltaT[pair][phiBin][thetaBin]; 00306 // Double_t corVal=grCor[pair]->Eval(dt); 00307 Double_t corVal=fastEvalForEvenSampling(grCor[pair],dt); 00308 corVal*=scale; 00309 Double_t binVal=histMap->GetBinContent(globalBin); 00310 histMap->SetBinContent(globalBin,binVal+corVal); 00311 } 00312 } 00313 } 00314 } 00315 if(fDebugMode) { 00316 char histName[180]; 00317 for(int i=0;i<fNumAnts;i++) { 00318 sprintf(histName,"grRaw%d_%d",i,counter); 00319 grRaw[i]->SetName(histName); 00320 grRaw[i]->SetTitle(histName); 00321 grRaw[i]->Write(); 00322 sprintf(histName,"grInt%d_%d",i,counter); 00323 grInt[i]->SetName(histName); 00324 grInt[i]->SetTitle(histName); 00325 grInt[i]->Write(); 00326 sprintf(histName,"grNorm%d_%d",i,counter); 00327 grNorm[i]->SetName(histName); 00328 grNorm[i]->SetTitle(histName); 00329 grNorm[i]->Write(); 00330 } 00331 for(int i=0;i<fNumPairs;i++) { 00332 sprintf(histName,"grCor%d_%d",i,counter); 00333 grCor[i]->SetName(histName); 00334 grCor[i]->SetTitle(histName); 00335 grCor[i]->Write(); 00336 } 00337 counter++; 00338 } 00339 for(int i=0;i<fNumAnts;i++) { 00340 if(grRaw[i]) delete grRaw[i]; 00341 if(grInt[i]) delete grInt[i]; 00342 if(grNorm[i]) delete grNorm[i]; 00343 } 00344 for(int i=0;i<fNumPairs;i++) { 00345 if(grCor[i]) delete grCor[i]; 00346 } 00347 //Moved the scaling to earlier for optimisation reasons 00348 // histMap->Scale(scale); 00349 return histMap; 00350 } 00351 00352 TH2D *AraEventCorrelator::getInterferometricMap(UsefulAtriStationEvent *evPtr, AraAntPol::AraAntPol_t polType,AraCorrelatorType::AraCorrelatorType_t corType) 00353 { 00354 static int counter=0; 00355 fillDeltaTArrays(corType); 00356 //Now need to get correlations for the fNumPairs antenna pairs and then look up the correlation values of each one 00357 Double_t scale=1./fNumPairs; 00358 TH2D *histMap = new TH2D("histMap","histMap",NUM_PHI_BINS,-180,180,NUM_THETA_BINS,-90,90); 00359 TGraph *grRaw[fNumAnts]; 00360 TGraph *grInt[fNumAnts]; 00361 TGraph *grNorm[fNumAnts]; 00362 TGraph *grCor[fNumPairs]; 00363 for(int i=0;i<fNumAnts;i++) { 00364 grRaw[i]=0; 00365 grInt[i]=0; 00366 grNorm[i]=0; 00367 } 00368 for(int i=0;i<fNumPairs;i++) 00369 grCor[i]=0; 00370 00371 if(polType==AraAntPol::kVertical) { 00372 for(int ind=0;ind<fNumAnts;ind++) { 00373 // std::cerr << ind << "\t" << fRfChanVPol[ind] << "\n"; 00374 grRaw[ind]=evPtr->getGraphFromRFChan(fRfChanVPol[ind]); 00375 grInt[ind]=FFTtools::getInterpolatedGraph(grRaw[ind],0.5); 00376 grNorm[ind]=getNormalisedGraph(grInt[ind]); 00377 } 00378 // std::cerr << "Got graphs and made int maps\n"; 00379 00380 for(int pair=0;pair<fNumPairs;pair++) { 00381 int ind1=0; 00382 int ind2=0; 00383 getPairIndices(pair,ind1,ind2); 00384 // std::cerr << pair << "\t" << ind1 << "\t" << ind2 << "\n"; 00385 00386 grCor[pair]=FFTtools::getCorrelationGraph(grNorm[ind1],grNorm[ind2]); 00387 for(int phiBin=0;phiBin<NUM_PHI_BINS;phiBin++) { 00388 for(int thetaBin=0;thetaBin<NUM_THETA_BINS;thetaBin++) { 00389 //I think this is the correct equation to work out the bin number 00390 //Could just use TH2::GetBin(binx,biny) but the below should be faster 00391 Int_t globalBin=(phiBin+1)+(thetaBin+1)*(NUM_PHI_BINS+2); 00392 Double_t dt=fVPolDeltaT[pair][phiBin][thetaBin]; 00393 //Double_t corVal=grCor[pair]->Eval(dt); 00394 Double_t corVal=fastEvalForEvenSampling(grCor[pair],dt); 00395 corVal*=scale; 00396 Double_t binVal=histMap->GetBinContent(globalBin); 00397 histMap->SetBinContent(globalBin,binVal+corVal); 00398 } 00399 } 00400 } 00401 } 00402 else { 00403 for(int ind=0;ind<fNumAnts;ind++) { 00404 grRaw[ind]=evPtr->getGraphFromRFChan(fRfChanHPol[ind]); 00405 grInt[ind]=FFTtools::getInterpolatedGraph(grRaw[ind],0.5); 00406 grNorm[ind]=getNormalisedGraph(grInt[ind]); 00407 } 00408 00409 for(int pair=0;pair<fNumPairs;pair++) { 00410 int ind1=0; 00411 int ind2=0; 00412 getPairIndices(pair,ind1,ind2); 00413 grCor[pair]=FFTtools::getCorrelationGraph(grNorm[ind1],grNorm[ind2]); 00414 for(int phiBin=0;phiBin<NUM_PHI_BINS;phiBin++) { 00415 for(int thetaBin=0;thetaBin<NUM_THETA_BINS;thetaBin++) { 00416 //I think this is the correct equation to work out the bin number 00417 //Could just use TH2::GetBin(binx,biny) but the below should be faster 00418 Int_t globalBin=(phiBin+1)+(thetaBin+1)*(NUM_PHI_BINS+2); 00419 Double_t dt=fHPolDeltaT[pair][phiBin][thetaBin]; 00420 // Double_t corVal=grCor[pair]->Eval(dt); 00421 Double_t corVal=fastEvalForEvenSampling(grCor[pair],dt); 00422 corVal*=scale; 00423 Double_t binVal=histMap->GetBinContent(globalBin); 00424 histMap->SetBinContent(globalBin,binVal+corVal); 00425 } 00426 } 00427 } 00428 } 00429 if(fDebugMode) { 00430 char histName[180]; 00431 for(int i=0;i<fNumAnts;i++) { 00432 sprintf(histName,"grRaw%d_%d",i,counter); 00433 grRaw[i]->SetName(histName); 00434 grRaw[i]->SetTitle(histName); 00435 grRaw[i]->Write(); 00436 sprintf(histName,"grInt%d_%d",i,counter); 00437 grInt[i]->SetName(histName); 00438 grInt[i]->SetTitle(histName); 00439 grInt[i]->Write(); 00440 sprintf(histName,"grNorm%d_%d",i,counter); 00441 grNorm[i]->SetName(histName); 00442 grNorm[i]->SetTitle(histName); 00443 grNorm[i]->Write(); 00444 } 00445 for(int i=0;i<fNumPairs;i++) { 00446 sprintf(histName,"grCor%d_%d",i,counter); 00447 grCor[i]->SetName(histName); 00448 grCor[i]->SetTitle(histName); 00449 grCor[i]->Write(); 00450 } 00451 counter++; 00452 } 00453 for(int i=0;i<fNumAnts;i++) { 00454 if(grRaw[i]) delete grRaw[i]; 00455 if(grInt[i]) delete grInt[i]; 00456 if(grNorm[i]) delete grNorm[i]; 00457 } 00458 for(int i=0;i<fNumPairs;i++) { 00459 if(grCor[i]) delete grCor[i]; 00460 } 00461 //Moved the scaling to earlier for optimisation reasons 00462 // histMap->Scale(scale); 00463 return histMap; 00464 } 00465 00466 TGraph* AraEventCorrelator::getNormalisedGraph(TGraph *grIn) 00467 { 00468 Double_t rms=grIn->GetRMS(2); 00469 Double_t mean=grIn->GetMean(2); 00470 Double_t *xVals = grIn->GetX(); 00471 Double_t *yVals = grIn->GetY(); 00472 Int_t numPoints = grIn->GetN(); 00473 Double_t *newY = new Double_t [numPoints]; 00474 for(int i=0;i<numPoints;i++) { 00475 newY[i]=(yVals[i]-mean)/rms; 00476 } 00477 TGraph *grOut = new TGraph(numPoints,xVals,newY); 00478 delete [] newY; 00479 return grOut; 00480 } 00481 00482 Double_t AraEventCorrelator::fastEvalForEvenSampling(TGraph *grIn, Double_t xvalue) 00483 { 00484 Int_t numPoints=grIn->GetN(); 00485 if(numPoints<2) return 0; 00486 Double_t *xVals=grIn->GetX(); 00487 Double_t *yVals=grIn->GetY(); 00488 Double_t dx=xVals[1]-xVals[0]; 00489 if(dx<=0) return 0; 00490 00491 Int_t p0=Int_t((xvalue-xVals[0])/dx); 00492 if(p0<0) p0=0; 00493 if(p0>=numPoints) p0=numPoints-2; 00494 return FFTtools::simpleInterploate(xVals[p0],yVals[p0],xVals[p0+1],yVals[p0+1],xvalue); 00495 }
Generated on Wed Aug 8 16:20:07 2012 for ARA ROOT Test Bed Software by
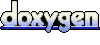