AraDisplay/AraTBCanvasMaker.cxx
00001 00002 00003 00004 00005 00006 00007 00008 #include <fstream> 00009 #include <iostream> 00010 #include "AraTBCanvasMaker.h" 00011 #include "AraGeomTool.h" 00012 #include "UsefulIcrrStationEvent.h" 00013 #include "AraWaveformGraph.h" 00014 #include "AraEventCorrelator.h" 00015 #include "AraFFTGraph.h" 00016 #include "araIcrrDefines.h" 00017 00018 00019 #include "TString.h" 00020 #include "TObjArray.h" 00021 #include "TObjString.h" 00022 #include "TVector3.h" 00023 #include "TROOT.h" 00024 #include "TPaveText.h" 00025 #include "TPad.h" 00026 #include "TText.h" 00027 #include "TLatex.h" 00028 #include "TGraph.h" 00029 #include "TStyle.h" 00030 #include "TCanvas.h" 00031 #include "TAxis.h" 00032 #include "TH1.h" 00033 #include "TH2.h" 00034 #include "TList.h" 00035 #include "TFile.h" 00036 #include "TObject.h" 00037 #include "TTimeStamp.h" 00038 #include "TGeoManager.h" 00039 #include "TGeoVolume.h" 00040 #include "TView3D.h" 00041 00042 #include "FFTtools.h" 00043 00044 00045 AraTBCanvasMaker* AraTBCanvasMaker::fgInstance = 0; 00046 AraGeomTool *fTBACMGeomTool=0; 00047 00048 00049 00050 00051 AraWaveformGraph *grTBElec[NUM_DIGITIZED_ICRR_CHANNELS]={0}; 00052 AraWaveformGraph *grTBElecFiltered[NUM_DIGITIZED_ICRR_CHANNELS]={0}; 00053 AraWaveformGraph *grTBElecHilbert[NUM_DIGITIZED_ICRR_CHANNELS]={0}; 00054 AraFFTGraph *grTBElecFFT[NUM_DIGITIZED_ICRR_CHANNELS]={0}; 00055 AraFFTGraph *grTBElecAveragedFFT[NUM_DIGITIZED_ICRR_CHANNELS]={0}; 00056 00057 AraWaveformGraph *grTBRFChan[MAX_RFCHANS_PER_ICRR]={0}; 00058 AraWaveformGraph *grTBRFChanFiltered[MAX_RFCHANS_PER_ICRR]={0}; 00059 AraWaveformGraph *grTBRFChanHilbert[MAX_RFCHANS_PER_ICRR]={0}; 00060 AraFFTGraph *grTBRFChanFFT[MAX_RFCHANS_PER_ICRR]={0}; 00061 AraFFTGraph *grTBRFChanAveragedFFT[MAX_RFCHANS_PER_ICRR]={0}; 00062 00063 00064 TH1D *AraTBCanvasMaker::getFFTHisto(int ant) 00065 { 00066 if(ant<0 || ant>=RFCHANS_PER_ICRR) return NULL; 00067 if(grTBRFChan[ant]) 00068 return grTBRFChan[ant]->getFFTHisto(); 00069 return NULL; 00070 00071 } 00072 00073 AraTBCanvasMaker::AraTBCanvasMaker(AraCalType::AraCalType_t calType) 00074 { 00075 //Default constructor 00076 fTBACMGeomTool=AraGeomTool::Instance(); 00077 fNumAntsInMap=4; 00078 fWebPlotterMode=0; 00079 fPassBandFilter=0; 00080 fNotchFilter=0; 00081 fLowPassEdge=200; 00082 fHighPassEdge=1200; 00083 fLowNotchEdge=235; 00084 fHighNotchEdge=500; 00085 fMinVoltLimit=-60; 00086 fMaxVoltLimit=60; 00087 fPhiMax=0; 00088 fMinClockVoltLimit=-200; 00089 fMaxClockVoltLimit=200; 00090 fAutoScale=1; 00091 fMinTimeLimit=0; 00092 fMaxTimeLimit=250; 00093 if(AraCalType::hasCableDelays(calType)) { 00094 fMinTimeLimit=-200; 00095 fMaxTimeLimit=150; 00096 } 00097 fMinPowerLimit=-60; 00098 fMaxPowerLimit=60; 00099 fMinFreqLimit=0; 00100 fMaxFreqLimit=1200; 00101 fWaveformOption=AraDisplayFormatOption::kWaveform; 00102 fRedoEventCanvas=0; 00103 //fRedoSurfCanvas=0; 00104 fLastWaveformFormat=AraDisplayFormatOption::kWaveform; 00105 fNewEvent=1; 00106 fCalType=calType; 00107 fCorType=AraCorrelatorType::kSphericalDist40; 00108 fgInstance=this; 00109 memset(grTBElec,0,sizeof(AraWaveformGraph*)*NUM_DIGITIZED_ICRR_CHANNELS); 00110 memset(grTBElecFiltered,0,sizeof(AraWaveformGraph*)*NUM_DIGITIZED_ICRR_CHANNELS); 00111 memset(grTBElecHilbert,0,sizeof(AraWaveformGraph*)*NUM_DIGITIZED_ICRR_CHANNELS); 00112 memset(grTBElecFFT,0,sizeof(AraFFTGraph*)*NUM_DIGITIZED_ICRR_CHANNELS); 00113 memset(grTBElecAveragedFFT,0,sizeof(AraFFTGraph*)*NUM_DIGITIZED_ICRR_CHANNELS); 00114 00115 memset(grTBRFChan,0,sizeof(AraWaveformGraph*)*MAX_RFCHANS_PER_ICRR); 00116 memset(grTBRFChanFiltered,0,sizeof(AraWaveformGraph*)*MAX_RFCHANS_PER_ICRR); 00117 memset(grTBRFChanHilbert,0,sizeof(AraWaveformGraph*)*MAX_RFCHANS_PER_ICRR); 00118 memset(grTBRFChanFFT,0,sizeof(AraFFTGraph*)*MAX_RFCHANS_PER_ICRR); 00119 memset(grTBRFChanAveragedFFT,0,sizeof(AraFFTGraph*)*MAX_RFCHANS_PER_ICRR); 00120 switch(fCalType) { 00121 case AraCalType::kNoCalib: 00122 fMaxVoltLimit=3000; 00123 fMinVoltLimit=1000; 00124 case AraCalType::kJustUnwrap: 00125 fMinTimeLimit=0; 00126 fMaxTimeLimit=260; 00127 break; 00128 default: 00129 break; 00130 } 00131 00132 00133 } 00134 00135 AraTBCanvasMaker::~AraTBCanvasMaker() 00136 { 00137 //Default destructor 00138 } 00139 00140 00141 00142 //______________________________________________________________________________ 00143 AraTBCanvasMaker* AraTBCanvasMaker::Instance() 00144 { 00145 //static function 00146 return (fgInstance) ? (AraTBCanvasMaker*) fgInstance : new AraTBCanvasMaker(); 00147 } 00148 00149 00150 TPad *AraTBCanvasMaker::getEventInfoCanvas(UsefulIcrrStationEvent *evPtr, TPad *useCan, Int_t runNumber) 00151 { 00152 static UInt_t lastEventNumber=0; 00153 static TPaveText *leftPave=0; 00154 static TPaveText *midLeftPave=0; 00155 static TPaveText *midRightPave=0; 00156 static TPaveText *rightPave=0; 00157 00158 00159 if(!fTBACMGeomTool) 00160 fTBACMGeomTool=AraGeomTool::Instance(); 00161 char textLabel[180]; 00162 TPad *topPad; 00163 if(!useCan) { 00164 topPad = new TPad("padEventInfo","padEventInfo",0.2,0.9,0.8,1); 00165 topPad->Draw(); 00166 } 00167 else { 00168 topPad=useCan; 00169 } 00170 if(1) { 00171 fNewEvent=1; 00172 topPad->Clear(); 00173 topPad->SetTopMargin(0.05); 00174 topPad->Divide(4,1); 00175 topPad->cd(1); 00176 if(leftPave) delete leftPave; 00177 leftPave = new TPaveText(0,0.1,1,0.9); 00178 leftPave->SetName("leftPave"); 00179 leftPave->SetBorderSize(0); 00180 leftPave->SetFillColor(0); 00181 leftPave->SetTextAlign(13); 00182 if(runNumber) { 00183 sprintf(textLabel,"Run: %d",runNumber); 00184 TText *runText = leftPave->AddText(textLabel); 00185 runText->SetTextColor(50); 00186 } 00187 if(evPtr->stationId==0) sprintf(textLabel,"TestBed Event: %d",evPtr->head.eventNumber); 00188 else if(evPtr->stationId==1) sprintf(textLabel,"Station1 Event: %d",evPtr->head.eventNumber); 00189 else sprintf(textLabel,"Event: %d",evPtr->head.eventNumber); 00190 00191 TText *eventText = leftPave->AddText(textLabel); 00192 eventText->SetTextColor(50); 00193 leftPave->Draw(); 00194 00195 00196 topPad->cd(2); 00197 gPad->SetRightMargin(0); 00198 gPad->SetLeftMargin(0); 00199 if(midLeftPave) delete midLeftPave; 00200 midLeftPave = new TPaveText(0,0.1,0.99,0.9); 00201 midLeftPave->SetName("midLeftPave"); 00202 midLeftPave->SetBorderSize(0); 00203 midLeftPave->SetTextAlign(13); 00204 TTimeStamp trigTime((time_t)evPtr->head.unixTime,(Int_t)1000*evPtr->head.unixTimeUs); 00205 sprintf(textLabel,"Time: %s",trigTime.AsString("s")); 00206 TText *timeText = midLeftPave->AddText(textLabel); 00207 timeText->SetTextColor(1); 00208 sprintf(textLabel,"Sub: %f",(trigTime.GetNanoSec()/1e9)); 00209 TText *timeText2 = midLeftPave->AddText(textLabel); 00210 timeText2->SetTextColor(1); 00211 midLeftPave->Draw(); 00212 // midLeftPave->Modified(); 00213 gPad->Modified(); 00214 gPad->Update(); 00215 00216 topPad->cd(3); 00217 if(midRightPave) delete midRightPave; 00218 midRightPave = new TPaveText(0,0.1,1,0.95); 00219 midRightPave->SetBorderSize(0); 00220 midRightPave->SetTextAlign(13); 00221 sprintf(textLabel,"PPS Num %d", 00222 evPtr->trig.ppsNum); 00223 midRightPave->AddText(textLabel); 00224 sprintf(textLabel,"Trig Type %d%d%d", 00225 evPtr->trig.isInTrigType(2), 00226 evPtr->trig.isInTrigType(1), 00227 evPtr->trig.isInTrigType(0)); 00228 midRightPave->AddText(textLabel); 00229 sprintf(textLabel,"Pattern %d%d%d%d%d%d%d%d", 00230 evPtr->trig.isInTrigPattern(7), 00231 evPtr->trig.isInTrigPattern(6), 00232 evPtr->trig.isInTrigPattern(5), 00233 evPtr->trig.isInTrigPattern(4), 00234 evPtr->trig.isInTrigPattern(3), 00235 evPtr->trig.isInTrigPattern(2), 00236 evPtr->trig.isInTrigPattern(1), 00237 evPtr->trig.isInTrigPattern(0)); 00238 midRightPave->AddText(textLabel); 00239 midRightPave->Draw(); 00240 00241 00242 topPad->cd(4); 00243 if(rightPave) delete rightPave; 00244 rightPave = new TPaveText(0,0.1,1,0.95); 00245 rightPave->SetBorderSize(0); 00246 rightPave->SetTextAlign(13); 00247 rightPave->Draw(); 00248 topPad->Update(); 00249 topPad->Modified(); 00250 00251 lastEventNumber=evPtr->head.eventNumber; 00252 } 00253 00254 return topPad; 00255 } 00256 00257 00258 TPad *AraTBCanvasMaker::quickGetEventViewerCanvasForWebPlottter(UsefulIcrrStationEvent *evPtr, TPad *useCan) 00259 { 00260 TPad *retCan=0; 00261 fWebPlotterMode=1; 00262 // static Int_t lastEventView=0; 00263 00264 if(fAutoScale) { 00265 fMinVoltLimit=1e9; 00266 fMaxVoltLimit=-1e9; 00267 fMinClockVoltLimit=1e9; 00268 fMaxClockVoltLimit=-1e9; 00269 } 00270 00271 00272 00273 for(int chan=0;chan<NUM_DIGITIZED_ICRR_CHANNELS;chan++) { 00274 if(grTBElec[chan]) delete grTBElec[chan]; 00275 if(grTBElecFFT[chan]) delete grTBElecFFT[chan]; 00276 if(grTBElecHilbert[chan]) delete grTBElecHilbert[chan]; 00277 grTBElec[chan]=0; 00278 grTBElecFFT[chan]=0; 00279 grTBElecHilbert[chan]=0; 00280 // if(grTBElecAveragedFFT[chan]) delete grTBElecAveragedFFT[chan]; 00281 00282 TGraph *grTemp = evPtr->getGraphFromElecChan(chan); 00283 grTBElec[chan] = new AraWaveformGraph(grTemp->GetN(),grTemp->GetX(),grTemp->GetY()); 00284 grTBElec[chan]->setElecChan(chan); 00285 // std::cout << evPtr->head.eventNumber << "\n"; 00286 // std::cout << surf << "\t" << chan << "\t" 00287 // << grTBElec[chan]->GetRMS(2) << std::endl; 00288 00289 if(fWaveformOption==AraDisplayFormatOption::kAveragedFFT) { 00290 TGraph *grTempFFT = grTBElec[chan]->getFFT(); 00291 grTBElecFFT[chan]=new AraFFTGraph(grTempFFT->GetN(), 00292 grTempFFT->GetX(), 00293 grTempFFT->GetY()); 00294 if(!grTBElecAveragedFFT[chan]) { 00295 grTBElecAveragedFFT[chan]=new AraFFTGraph(grTempFFT->GetN(), 00296 grTempFFT->GetX(), 00297 grTempFFT->GetY()); 00298 } 00299 else { 00300 grTBElecAveragedFFT[chan]->AddFFT(grTBElecFFT[chan]); 00301 } 00302 delete grTempFFT; 00303 } 00304 00305 00306 if(fAutoScale) { 00307 Int_t numPoints=grTemp->GetN(); 00308 Double_t *yVals=grTemp->GetY(); 00309 00310 for(int i=0;i<numPoints;i++) { 00311 if(yVals[i]<fMinVoltLimit) 00312 fMinVoltLimit=yVals[i]; 00313 if(yVals[i]>fMaxVoltLimit) 00314 fMaxVoltLimit=yVals[i]; 00315 } 00316 } 00317 00318 delete grTemp; 00319 } 00320 00321 00322 00323 for(int rfchan=0;rfchan<RFCHANS_PER_ICRR;rfchan++) { 00324 if(grTBRFChan[rfchan]) delete grTBRFChan[rfchan]; 00325 if(grTBRFChanFFT[rfchan]) delete grTBRFChanFFT[rfchan]; 00326 if(grTBRFChanHilbert[rfchan]) delete grTBRFChanHilbert[rfchan]; 00327 grTBRFChan[rfchan]=0; 00328 grTBRFChanFFT[rfchan]=0; 00329 grTBRFChanHilbert[rfchan]=0; 00330 // if(grTBRFChanAveragedFFT[chan]) delete grTBRFChanAveragedFFT[chan]; 00331 //Need to work out how to do this 00332 TGraph *grTemp = evPtr->getGraphFromRFChan(rfchan); 00333 grTBRFChan[rfchan] = new AraWaveformGraph(grTemp->GetN(),grTemp->GetX(),grTemp->GetY()); 00334 grTBRFChan[rfchan]->setRFChan(rfchan, evPtr->stationId); 00335 // std::cout << evPtr->head.eventNumber << "\n"; 00336 // std::cout << surf << "\t" << chan << "\t" 00337 // << grTBElec[chan]->GetRMS(2) << std::endl; 00338 00339 00340 if(fWaveformOption==AraDisplayFormatOption::kAveragedFFT) { 00341 TGraph *grTempFFT = grTBRFChan[rfchan]->getFFT(); 00342 grTBRFChanFFT[rfchan]=new AraFFTGraph(grTempFFT->GetN(), 00343 grTempFFT->GetX(), 00344 grTempFFT->GetY()); 00345 if(!grTBRFChanAveragedFFT[rfchan]) { 00346 grTBRFChanAveragedFFT[rfchan]=new AraFFTGraph(grTempFFT->GetN(), 00347 grTempFFT->GetX(), 00348 grTempFFT->GetY()); 00349 } 00350 else { 00351 grTBRFChanAveragedFFT[rfchan]->AddFFT(grTBRFChanFFT[rfchan]); 00352 } 00353 delete grTempFFT; 00354 } 00355 00356 00357 00358 if(fAutoScale) { 00359 Int_t numPoints=grTemp->GetN(); 00360 Double_t *yVals=grTemp->GetY(); 00361 00362 for(int i=0;i<numPoints;i++) { 00363 if(yVals[i]<fMinVoltLimit) 00364 fMinVoltLimit=yVals[i]; 00365 if(yVals[i]>fMaxVoltLimit) 00366 fMaxVoltLimit=yVals[i]; 00367 } 00368 } 00369 delete grTemp; 00370 } 00371 00372 00373 if(fAutoScale) { 00374 if(fCalType!=AraCalType::kNoCalib && fCalType!=AraCalType::kJustUnwrap) { 00375 if(fMaxVoltLimit>-1*fMinVoltLimit) { 00376 fMinVoltLimit=-1*fMaxVoltLimit; 00377 } 00378 else { 00379 fMaxVoltLimit=-1*fMinVoltLimit; 00380 } 00381 } 00382 00383 00384 if(fMaxClockVoltLimit>-1*fMinClockVoltLimit) { 00385 fMinClockVoltLimit=-1*fMaxClockVoltLimit; 00386 } 00387 else { 00388 fMaxClockVoltLimit=-1*fMinClockVoltLimit; 00389 } 00390 } 00391 00392 00393 fRedoEventCanvas=0; 00394 00395 retCan=AraTBCanvasMaker::getCanvasForWebPlotter(evPtr,useCan); 00396 00397 00398 00399 return retCan; 00400 00401 } 00402 00403 TPad *AraTBCanvasMaker::getEventViewerCanvas(UsefulIcrrStationEvent *evPtr, 00404 TPad *useCan) 00405 { 00406 TPad *retCan=0; 00407 00408 static UInt_t lastEventNumber=0; 00409 00410 if(fAutoScale) { 00411 fMinVoltLimit=1e9; 00412 fMaxVoltLimit=-1e9; 00413 fMinClockVoltLimit=0; 00414 fMaxClockVoltLimit=0; 00415 } 00416 00417 00418 00419 for(int chan=0;chan<NUM_DIGITIZED_ICRR_CHANNELS;chan++) { 00420 if(grTBElec[chan]) delete grTBElec[chan]; 00421 if(grTBElecFFT[chan]) delete grTBElecFFT[chan]; 00422 if(grTBElecHilbert[chan]) delete grTBElecHilbert[chan]; 00423 grTBElec[chan]=0; 00424 grTBElecFFT[chan]=0; 00425 grTBElecHilbert[chan]=0; 00426 00427 TGraph *grTemp = evPtr->getGraphFromElecChan(chan); 00428 grTBElec[chan] = new AraWaveformGraph(grTemp->GetN(),grTemp->GetX(),grTemp->GetY()); 00429 grTBElec[chan]->setElecChan(chan); 00430 00431 00432 if(fWaveformOption==AraDisplayFormatOption::kAveragedFFT) { 00433 TGraph *grTempFFT = grTBElec[chan]->getFFT(); 00434 grTBElecFFT[chan]=new AraFFTGraph(grTempFFT->GetN(), 00435 grTempFFT->GetX(), 00436 grTempFFT->GetY()); 00437 if(!grTBElecAveragedFFT[chan]) { 00438 grTBElecAveragedFFT[chan]=new AraFFTGraph(grTempFFT->GetN(), 00439 grTempFFT->GetX(), 00440 grTempFFT->GetY()); 00441 } 00442 else { 00443 grTBElecAveragedFFT[chan]->AddFFT(grTBElecFFT[chan]); 00444 } 00445 delete grTempFFT; 00446 } 00447 00448 00449 if(fAutoScale) { 00450 Int_t numPoints=grTemp->GetN(); 00451 Double_t *yVals=grTemp->GetY(); 00452 00453 if(chan%9==8) { 00454 //Clock channel 00455 for(int i=0;i<numPoints;i++) { 00456 if(yVals[i]<fMinClockVoltLimit) 00457 fMinClockVoltLimit=yVals[i]; 00458 if(yVals[i]>fMaxClockVoltLimit) 00459 fMaxClockVoltLimit=yVals[i]; 00460 } 00461 } 00462 else{ 00463 for(int i=0;i<numPoints;i++) { 00464 if(yVals[i]<fMinVoltLimit) 00465 fMinVoltLimit=yVals[i]; 00466 if(yVals[i]>fMaxVoltLimit) 00467 fMaxVoltLimit=yVals[i]; 00468 } 00469 } 00470 } 00471 00472 delete grTemp; 00473 } 00474 // std::cout << "Limits\t" << fMinVoltLimit << "\t" << fMaxVoltLimit << "\n"; 00475 00476 00477 for(int rfchan=0;rfchan<(evPtr->numRFChans);rfchan++) { 00478 if(grTBRFChan[rfchan]) delete grTBRFChan[rfchan]; 00479 if(grTBRFChanFFT[rfchan]) delete grTBRFChanFFT[rfchan]; 00480 if(grTBRFChanHilbert[rfchan]) delete grTBRFChanHilbert[rfchan]; 00481 grTBRFChan[rfchan]=0; 00482 grTBRFChanFFT[rfchan]=0; 00483 grTBRFChanHilbert[rfchan]=0; 00484 //Need to work out how to do this 00485 TGraph *grTemp = evPtr->getGraphFromRFChan(rfchan); 00486 grTBRFChan[rfchan] = new AraWaveformGraph(grTemp->GetN(),grTemp->GetX(),grTemp->GetY()); 00487 00488 00489 00490 grTBRFChan[rfchan]->setRFChan(rfchan, evPtr->stationId); 00491 // std::cout << evPtr->head.eventNumber << "\n"; 00492 // std::cout << surf << "\t" << chan << "\t" 00493 // << grTBElec[chan]->GetRMS(2) << std::endl; 00494 00495 if(fWaveformOption==AraDisplayFormatOption::kAveragedFFT) { 00496 TGraph *grTempFFT = grTBRFChan[rfchan]->getFFT(); 00497 grTBRFChanFFT[rfchan]=new AraFFTGraph(grTempFFT->GetN(), 00498 grTempFFT->GetX(), 00499 grTempFFT->GetY()); 00500 if(!grTBRFChanAveragedFFT[rfchan]) { 00501 grTBRFChanAveragedFFT[rfchan]=new AraFFTGraph(grTempFFT->GetN(), 00502 grTempFFT->GetX(), 00503 grTempFFT->GetY()); 00504 } 00505 else { 00506 grTBRFChanAveragedFFT[rfchan]->AddFFT(grTBRFChanFFT[rfchan]); 00507 } 00508 delete grTempFFT; 00509 } 00510 if(fAutoScale) { 00511 Int_t numPoints=grTemp->GetN(); 00512 Double_t *yVals=grTemp->GetY(); 00513 00514 for(int i=0;i<numPoints;i++) { 00515 if(yVals[i]<fMinVoltLimit) 00516 fMinVoltLimit=yVals[i]; 00517 if(yVals[i]>fMaxVoltLimit) 00518 fMaxVoltLimit=yVals[i]; 00519 } 00520 } 00521 delete grTemp; 00522 } 00523 00524 if(fAutoScale) { 00525 00526 if(fCalType!=AraCalType::kNoCalib && fCalType!=AraCalType::kJustUnwrap) { 00527 if(fMaxVoltLimit>-1*fMinVoltLimit) { 00528 fMinVoltLimit=-1*fMaxVoltLimit; 00529 } 00530 else { 00531 fMaxVoltLimit=-1*fMinVoltLimit; 00532 } 00533 if(fMaxClockVoltLimit>-1*fMinClockVoltLimit) { 00534 fMinClockVoltLimit=-1*fMaxClockVoltLimit; 00535 } 00536 else { 00537 fMaxClockVoltLimit=-1*fMinClockVoltLimit; 00538 } 00539 } 00540 } 00541 00542 // std::cout << "Limits\t" << fMinVoltLimit << "\t" << fMaxVoltLimit << "\n"; 00543 00544 00545 fRedoEventCanvas=0; 00546 fNewEvent=0; 00547 00548 fRedoEventCanvas=0; 00549 if(fLastWaveformFormat!=fWaveformOption) fRedoEventCanvas=1; 00550 00551 00552 if(fCanvasLayout==AraDisplayCanvasLayoutOption::kElectronicsView) { 00553 retCan=AraTBCanvasMaker::getElectronicsCanvas(evPtr,useCan); 00554 } 00555 else if(fCanvasLayout==AraDisplayCanvasLayoutOption::kRFChanView) { 00556 retCan=AraTBCanvasMaker::getRFChannelCanvas(evPtr,useCan); 00557 } 00558 else if(fCanvasLayout==AraDisplayCanvasLayoutOption::kAntennaView) { 00559 retCan=AraTBCanvasMaker::getAntennaCanvas(evPtr,useCan); 00560 } 00561 else if(fCanvasLayout==AraDisplayCanvasLayoutOption::kIntMapView) { 00562 retCan=AraTBCanvasMaker::getIntMapCanvas(evPtr,useCan); 00563 } 00564 00565 00566 fLastWaveformFormat=fWaveformOption; 00567 fLastCanvasView=fCanvasLayout; 00568 00569 return retCan; 00570 00571 } 00572 00573 00574 TPad *AraTBCanvasMaker::getElectronicsCanvas(UsefulIcrrStationEvent *evPtr,TPad *useCan) 00575 { 00576 // gStyle->SetTitleH(0.1); 00577 gStyle->SetOptTitle(0); 00578 00579 if(!fTBACMGeomTool) 00580 fTBACMGeomTool=AraGeomTool::Instance(); 00581 char textLabel[180]; 00582 char padName[180]; 00583 TPad *canElec=0; 00584 TPad *plotPad=0; 00585 if(!useCan) { 00586 canElec = (TPad*) gROOT->FindObject("canElec"); 00587 if(!canElec) { 00588 canElec = new TCanvas("canElec","canElec",1000,600); 00589 } 00590 canElec->Clear(); 00591 canElec->SetTopMargin(0); 00592 TPaveText *leftPave = new TPaveText(0.05,0.92,0.95,0.98); 00593 leftPave->SetBorderSize(0); 00594 sprintf(textLabel," Event %d",evPtr->head.eventNumber); 00595 TText *eventText = leftPave->AddText(textLabel); 00596 eventText->SetTextColor(50); 00597 leftPave->Draw(); 00598 plotPad = new TPad("canElecMain","canElecMain",0,0,1,0.9); 00599 plotPad->Draw(); 00600 } 00601 else { 00602 plotPad=useCan; 00603 } 00604 plotPad->cd(); 00605 setupElecPadWithFrames(plotPad); 00606 00607 00608 00609 int count=0; 00610 00611 // A 00612 // 1 3 5 7 9 00613 // 2 4 6 8 00614 // B 00615 // 1 3 5 7 9 00616 // 2 4 6 8 00617 // C 00618 // 1 3 5 7 9 00619 // 2 4 6 8 00620 00621 for(int row=0;row<6;row++) { 00622 for(int column=0;column<5;column++) { 00623 plotPad->cd(); 00624 int labChip=(row/2); //0, 1 or 2 00625 int channel=(row%2)+2*column; 00626 int chanIndex=channel+9*labChip; 00627 if(channel>8) continue; 00628 00629 sprintf(padName,"elecChanPad%d",column+row*5); 00630 // std::cout << chanIndex << "\t" << labChip << "\t" << channel << "\t" << padName << "\n"; 00631 TPad *paddy1 = (TPad*) plotPad->FindObject(padName); 00632 paddy1->SetEditable(kTRUE); 00633 deleteTGraphsFromElecPad(paddy1,chanIndex); 00634 00635 00636 00637 if(fWaveformOption==AraDisplayFormatOption::kPowerSpectralDensity){ 00638 if(!grTBElecFFT[chanIndex]) { 00639 TGraph *grTemp=grTBElec[chanIndex]->getFFT(); 00640 grTBElecFFT[chanIndex]=new AraFFTGraph(grTemp->GetN(),grTemp->GetX(),grTemp->GetY()); 00641 delete grTemp; 00642 } 00643 grTBElecFFT[chanIndex]->Draw("l"); 00644 } 00645 else if(fWaveformOption==AraDisplayFormatOption::kAveragedFFT){ 00646 grTBElecAveragedFFT[chanIndex]->Draw("l"); 00647 } 00648 else if(fWaveformOption==AraDisplayFormatOption::kHilbertEnvelope) { 00649 if(!grTBElecHilbert[chanIndex]) { 00650 TGraph *grTemp=grTBElec[chanIndex]->getHilbert(); 00651 grTBElecHilbert[chanIndex]=new AraWaveformGraph(grTemp->GetN(),grTemp->GetX(),grTemp->GetY()); 00652 delete grTemp; 00653 } 00654 grTBElecHilbert[chanIndex]->Draw("l"); 00655 } 00656 else if(fWaveformOption==AraDisplayFormatOption::kWaveform){ 00657 00658 grTBElec[chanIndex]->Draw("l"); 00659 00660 00661 if(fAutoScale) { 00662 TList *listy = gPad->GetListOfPrimitives(); 00663 for(int i=0;i<listy->GetSize();i++) { 00664 TObject *fred = listy->At(i); 00665 TH1F *tempHist = (TH1F*) fred; 00666 if(tempHist->InheritsFrom("TH1")) { 00667 if(channel<8) { 00668 tempHist->GetYaxis()->SetRangeUser(fMinVoltLimit,fMaxVoltLimit); 00669 } 00670 else { 00671 tempHist->GetYaxis()->SetRangeUser(fMinClockVoltLimit,fMaxClockVoltLimit); 00672 } 00673 } 00674 } 00675 } 00676 } 00677 00678 00679 count++; 00680 paddy1->SetEditable(kFALSE); 00681 } 00682 } 00683 00684 if(!useCan) 00685 return canElec; 00686 else 00687 return plotPad; 00688 00689 00690 } 00691 00692 00693 TPad *AraTBCanvasMaker::getCanvasForWebPlotter(UsefulIcrrStationEvent *evPtr, 00694 TPad *useCan) 00695 { 00696 // gStyle->SetTitleH(0.1); 00697 gStyle->SetOptTitle(0); 00698 00699 if(!fTBACMGeomTool) 00700 fTBACMGeomTool=AraGeomTool::Instance(); 00701 char textLabel[180]; 00702 char padName[180]; 00703 TPad *canRFChan=0; 00704 TPad *plotPad=0; 00705 if(!useCan) { 00706 canRFChan = (TPad*) gROOT->FindObject("canRFChan"); 00707 if(!canRFChan) { 00708 canRFChan = new TCanvas("canRFChan","canRFChan",1000,600); 00709 } 00710 canRFChan->Clear(); 00711 canRFChan->SetTopMargin(0); 00712 TPaveText *leftPave = new TPaveText(0.05,0.92,0.95,0.98); 00713 leftPave->SetBorderSize(0); 00714 sprintf(textLabel,"Event %d",evPtr->head.eventNumber); 00715 TText *eventText = leftPave->AddText(textLabel); 00716 eventText->SetTextColor(50); 00717 leftPave->Draw(); 00718 plotPad = new TPad("canRFChanMain","canRFChanMain",0,0,1,0.9); 00719 plotPad->Draw(); 00720 } 00721 else { 00722 plotPad=useCan; 00723 } 00724 plotPad->cd(); 00725 setupRFChanPadWithFrames(plotPad, evPtr->stationId); 00726 00727 00728 00729 int count=0; 00730 00731 00732 // 1 2 3 4 00733 // 5 6 7 8 00734 // 9 10 11 12 00735 // 13 14 15 16 00736 00737 for(int column=0;column<4;column++) { 00738 for(int row=0;row<4;row++) { 00739 plotPad->cd(); 00740 int rfChan=column+4*row; 00741 00742 sprintf(padName,"rfChanPad%d",column+4*row); 00743 TPad *paddy1 = (TPad*) plotPad->FindObject(padName); 00744 paddy1->SetEditable(kTRUE); 00745 deleteTGraphsFromRFPad(paddy1,rfChan); 00746 paddy1->cd(); 00747 00748 grTBRFChan[rfChan]->Draw("l"); 00749 00750 if(fAutoScale) { 00751 TList *listy = gPad->GetListOfPrimitives(); 00752 for(int i=0;i<listy->GetSize();i++) { 00753 TObject *fred = listy->At(i); 00754 TH1F *tempHist = (TH1F*) fred; 00755 if(tempHist->InheritsFrom("TH1")) { 00756 tempHist->GetYaxis()->SetRangeUser(fMinVoltLimit,fMaxVoltLimit); 00757 00758 } 00759 } 00760 } 00761 00762 00763 count++; 00764 paddy1->SetEditable(kFALSE); 00765 } 00766 } 00767 00768 if(!useCan) 00769 return canRFChan; 00770 else 00771 return plotPad; 00772 00773 00774 } 00775 00776 TPad *AraTBCanvasMaker::getRFChannelCanvas(UsefulIcrrStationEvent *evPtr, 00777 TPad *useCan) 00778 { 00779 // gStyle->SetTitleH(0.1); 00780 gStyle->SetOptTitle(0); 00781 00782 if(!fTBACMGeomTool) 00783 fTBACMGeomTool=AraGeomTool::Instance(); 00784 char textLabel[180]; 00785 char padName[180]; 00786 TPad *canRFChan=0; 00787 TPad *plotPad=0; 00788 if(!useCan) { 00789 canRFChan = (TPad*) gROOT->FindObject("canRFChan"); 00790 if(!canRFChan) { 00791 canRFChan = new TCanvas("canRFChan","canRFChan",1000,600); 00792 } 00793 canRFChan->Clear(); 00794 canRFChan->SetTopMargin(0); 00795 TPaveText *leftPave = new TPaveText(0.05,0.92,0.95,0.98); 00796 leftPave->SetBorderSize(0); 00797 sprintf(textLabel,"Event %d",evPtr->head.eventNumber); 00798 TText *eventText = leftPave->AddText(textLabel); 00799 eventText->SetTextColor(50); 00800 leftPave->Draw(); 00801 plotPad = new TPad("canRFChanMain","canRFChanMain",0,0,1,0.9); 00802 plotPad->Draw(); 00803 } 00804 else { 00805 plotPad=useCan; 00806 } 00807 plotPad->cd(); 00808 setupRFChanPadWithFrames(plotPad, evPtr->stationId); 00809 int maxColumns=0; 00810 int maxRows=0; 00811 00812 if(evPtr->stationId==1){ 00813 maxColumns=4; 00814 maxRows=5; 00815 } 00816 else { 00817 maxColumns=4; 00818 maxRows=4; 00819 } 00820 00821 00822 for(int column=0;column<maxColumns;column++) { 00823 for(int row=0;row<maxRows;row++) { 00824 plotPad->cd(); 00825 int rfChan=column+4*row; 00826 00827 sprintf(padName,"rfChanPad%d",column+4*row); 00828 TPad *paddy1 = (TPad*) plotPad->FindObject(padName); 00829 paddy1->SetEditable(kTRUE); 00830 deleteTGraphsFromRFPad(paddy1,rfChan); 00831 paddy1->cd(); 00832 00833 if(fWaveformOption==AraDisplayFormatOption::kPowerSpectralDensity){ 00834 if(!grTBRFChanFFT[rfChan]) { 00835 TGraph *grTemp=grTBRFChan[rfChan]->getFFT(); 00836 grTBRFChanFFT[rfChan]=new AraFFTGraph(grTemp->GetN(),grTemp->GetX(),grTemp->GetY()); 00837 delete grTemp; 00838 } 00839 grTBRFChanFFT[rfChan]->Draw("l"); 00840 } 00841 else if(fWaveformOption==AraDisplayFormatOption::kAveragedFFT){ 00842 grTBRFChanAveragedFFT[rfChan]->Draw("l"); 00843 } 00844 else if(fWaveformOption==AraDisplayFormatOption::kHilbertEnvelope) { 00845 if(!grTBRFChanHilbert[rfChan]) { 00846 TGraph *grTemp=grTBRFChan[rfChan]->getHilbert(); 00847 grTBRFChanHilbert[rfChan]=new AraWaveformGraph(grTemp->GetN(),grTemp->GetX(),grTemp->GetY()); 00848 delete grTemp; 00849 } 00850 grTBRFChanHilbert[rfChan]->Draw("l"); 00851 } 00852 else if(fWaveformOption==AraDisplayFormatOption::kWaveform) { 00853 grTBRFChan[rfChan]->Draw("l"); 00854 00855 if(fAutoScale) { 00856 TList *listy = gPad->GetListOfPrimitives(); 00857 for(int i=0;i<listy->GetSize();i++) { 00858 TObject *fred = listy->At(i); 00859 TH1F *tempHist = (TH1F*) fred; 00860 if(tempHist->InheritsFrom("TH1")) { 00861 tempHist->GetYaxis()->SetRangeUser(fMinVoltLimit,fMaxVoltLimit); 00862 } 00863 } 00864 } 00865 } 00866 00867 paddy1->SetEditable(kFALSE); 00868 } 00869 } 00870 00871 00872 if(!useCan) 00873 return canRFChan; 00874 else 00875 return plotPad; 00876 00877 } 00878 00879 //jpd this is the next one to change 00880 TPad *AraTBCanvasMaker::getAntennaCanvas(UsefulIcrrStationEvent *evPtr, 00881 TPad *useCan) 00882 { 00883 // gStyle->SetTitleH(0.1); 00884 gStyle->SetOptTitle(0); 00885 00886 if(!fTBACMGeomTool) 00887 fTBACMGeomTool=AraGeomTool::Instance(); 00888 char textLabel[180]; 00889 char padName[180]; 00890 TPad *canRFChan=0; 00891 TPad *plotPad=0; 00892 if(!useCan) { 00893 canRFChan = (TPad*) gROOT->FindObject("canRFChan"); 00894 if(!canRFChan) { 00895 canRFChan = new TCanvas("canRFChan","canRFChan",1000,600); 00896 } 00897 canRFChan->Clear(); 00898 canRFChan->SetTopMargin(0); 00899 TPaveText *leftPave = new TPaveText(0.05,0.92,0.95,0.98); 00900 leftPave->SetBorderSize(0); 00901 sprintf(textLabel,"Event %d",evPtr->head.eventNumber); 00902 TText *eventText = leftPave->AddText(textLabel); 00903 eventText->SetTextColor(50); 00904 leftPave->Draw(); 00905 plotPad = new TPad("canRFChanMain","canRFChanMain",0,0,1,0.9); 00906 plotPad->Draw(); 00907 } 00908 else { 00909 plotPad=useCan; 00910 } 00911 plotPad->cd(); 00912 setupAntPadWithFrames(plotPad, evPtr->stationId); 00913 00914 // int rfChanMap[4][4]={ 00915 AraAntPol::AraAntPol_t polMap[4][4]={{AraAntPol::kVertical,AraAntPol::kVertical,AraAntPol::kVertical,AraAntPol::kVertical}, 00916 {AraAntPol::kVertical,AraAntPol::kVertical,AraAntPol::kSurface,AraAntPol::kSurface}, 00917 {AraAntPol::kHorizontal,AraAntPol::kHorizontal,AraAntPol::kHorizontal,AraAntPol::kHorizontal}, 00918 {AraAntPol::kHorizontal,AraAntPol::kHorizontal,AraAntPol::kHorizontal,AraAntPol::kHorizontal}}; 00919 int antPolNumMap[4][4]={{0,1,2,3},{4,5,0,1},{0,1,2,3},{4,5,6,7}}; 00920 00921 00922 00923 00924 for(int row=0;row<4;row++) { 00925 for(int column=0;column<4;column++) { 00926 plotPad->cd(); 00927 int rfChan=fTBACMGeomTool->getRFChanByPolAndAnt(polMap[row][column],antPolNumMap[row][column], evPtr->stationId); 00928 // std::cout << row << "\t" << column << "\t" << rfChan << "\n"; 00929 00930 sprintf(padName,"antPad%d_%d",column,row); 00931 TPad *paddy1 = (TPad*) plotPad->FindObject(padName); 00932 paddy1->SetEditable(kTRUE); 00933 deleteTGraphsFromRFPad(paddy1,rfChan); 00934 paddy1->cd(); 00935 00936 if(fWaveformOption==AraDisplayFormatOption::kPowerSpectralDensity){ 00937 if(!grTBRFChanFFT[rfChan]) { 00938 TGraph *grTemp=grTBRFChan[rfChan]->getFFT(); 00939 grTBRFChanFFT[rfChan]=new AraFFTGraph(grTemp->GetN(),grTemp->GetX(),grTemp->GetY()); 00940 delete grTemp; 00941 } 00942 grTBRFChanFFT[rfChan]->Draw("l"); 00943 } 00944 else if(fWaveformOption==AraDisplayFormatOption::kAveragedFFT){ 00945 grTBRFChanAveragedFFT[rfChan]->Draw("l"); 00946 } 00947 else if(fWaveformOption==AraDisplayFormatOption::kHilbertEnvelope) { 00948 if(!grTBRFChanHilbert[rfChan]) { 00949 TGraph *grTemp=grTBRFChan[rfChan]->getHilbert(); 00950 grTBRFChanHilbert[rfChan]=new AraWaveformGraph(grTemp->GetN(),grTemp->GetX(),grTemp->GetY()); 00951 delete grTemp; 00952 } 00953 grTBRFChanHilbert[rfChan]->Draw("l"); 00954 } 00955 else if(fWaveformOption==AraDisplayFormatOption::kWaveform) { 00956 grTBRFChan[rfChan]->Draw("l"); 00957 00958 if(fAutoScale) { 00959 TList *listy = gPad->GetListOfPrimitives(); 00960 for(int i=0;i<listy->GetSize();i++) { 00961 TObject *fred = listy->At(i); 00962 TH1F *tempHist = (TH1F*) fred; 00963 if(tempHist->InheritsFrom("TH1")) { 00964 tempHist->GetYaxis()->SetRangeUser(fMinVoltLimit,fMaxVoltLimit); 00965 } 00966 } 00967 } 00968 } 00969 00970 paddy1->SetEditable(kFALSE); 00971 } 00972 } 00973 00974 00975 if(!useCan) 00976 return canRFChan; 00977 else 00978 return plotPad; 00979 00980 } 00981 00982 00983 TPad *AraTBCanvasMaker::getIntMapCanvas(UsefulIcrrStationEvent *evPtr, 00984 TPad *useCan) 00985 { 00986 static UInt_t lastEventNumber=0; 00987 static UInt_t lastUnixTime=0; 00988 static UInt_t lastUnixTimeUs=0; 00989 Int_t sameEvent=0; 00990 if(lastEventNumber==evPtr->head.eventNumber) { 00991 if(lastUnixTime==evPtr->head.unixTime) { 00992 if(lastUnixTimeUs==evPtr->head.unixTimeUs) { 00993 sameEvent=1; 00994 } 00995 } 00996 } 00997 lastEventNumber=evPtr->head.eventNumber; 00998 lastUnixTime=evPtr->head.unixTime; 00999 lastUnixTimeUs=evPtr->head.unixTimeUs; 01000 01001 01002 // gStyle->SetTitleH(0.1); 01003 gStyle->SetOptTitle(0); 01004 01005 if(!fTBACMGeomTool) 01006 fTBACMGeomTool=AraGeomTool::Instance(); 01007 char textLabel[180]; 01008 char padName[180]; 01009 TPad *canIntMap=0; 01010 TPad *plotPad=0; 01011 if(!useCan) { 01012 canIntMap = (TPad*) gROOT->FindObject("canIntMap"); 01013 if(!canIntMap) { 01014 canIntMap = new TCanvas("canIntMap","canIntMap",1000,600); 01015 } 01016 canIntMap->Clear(); 01017 canIntMap->SetTopMargin(0); 01018 TPaveText *leftPave = new TPaveText(0.05,0.92,0.95,0.98); 01019 leftPave->SetBorderSize(0); 01020 sprintf(textLabel,"Event %d",evPtr->head.eventNumber); 01021 TText *eventText = leftPave->AddText(textLabel); 01022 eventText->SetTextColor(50); 01023 leftPave->Draw(); 01024 plotPad = new TPad("canIntMapMain","canIntMapMain",0,0,1,0.9); 01025 plotPad->Draw(); 01026 } 01027 else { 01028 plotPad=useCan; 01029 } 01030 plotPad->cd(); 01031 plotPad->Clear(); 01032 AraEventCorrelator *araCorPtr = AraEventCorrelator::Instance(fNumAntsInMap, evPtr->stationId); 01033 static TH2D* histMapH=0; 01034 static TH2D* histMapV=0; 01035 plotPad->Divide(1,2); 01036 plotPad->cd(1); 01037 01038 if(histMapV) { 01039 if(!sameEvent) { 01040 delete histMapV; 01041 histMapV=0; 01042 } 01043 } 01044 01045 01046 if(!histMapV) 01047 histMapV =araCorPtr->getInterferometricMap(evPtr,AraAntPol::kVertical,fCorType); 01048 histMapV->SetName("histMapV"); 01049 histMapV->SetTitle("Vertical Polarisation"); 01050 histMapV->SetXTitle("Azimuth (Degrees)"); 01051 histMapV->SetYTitle("Elevation (Degrees)"); 01052 histMapV->SetStats(0); 01053 // histMapV->SetMaximum(1); 01054 // histMapV->SetMinimum(-1); 01055 histMapV->Draw("colz"); 01056 01057 plotPad->cd(2); 01058 if(histMapH) { 01059 if(!sameEvent) { 01060 delete histMapH; 01061 histMapH=0; 01062 } 01063 } 01064 if(!histMapH) 01065 histMapH =araCorPtr->getInterferometricMap(evPtr,AraAntPol::kHorizontal,fCorType); 01066 histMapH->SetName("histMapH"); 01067 histMapH->SetTitle("Hertical Polarisation"); 01068 histMapH->SetXTitle("Azimuth (Degrees)"); 01069 histMapH->SetYTitle("Elevation (Degrees)"); 01070 histMapH->SetStats(0); 01071 // histMapH->SetMaximum(1); 01072 // histMapH->SetMinimum(-1); 01073 histMapH->Draw("colz"); 01074 01075 01076 01077 01078 01079 if(!useCan) 01080 return canIntMap; 01081 else 01082 return plotPad; 01083 01084 } 01085 01086 01087 01088 01089 01090 01091 01092 void AraTBCanvasMaker::setupElecPadWithFrames(TPad *plotPad) 01093 { 01094 char textLabel[180]; 01095 char padName[180]; 01096 plotPad->cd(); 01097 if(fLastCanvasView!=AraDisplayCanvasLayoutOption::kElectronicsView) { 01098 plotPad->Clear(); 01099 } 01100 if(fRedoEventCanvas){ 01101 plotPad->Clear(); 01102 } 01103 01104 fLastCanvasView=AraDisplayCanvasLayoutOption::kElectronicsView; 01105 01106 static int elecPadsDone=0; 01107 if(elecPadsDone && !fRedoEventCanvas) { 01108 int errors=0; 01109 for(int i=0;i<30;i++) { 01110 sprintf(padName,"elecChanPad%d",i); 01111 TPad *paddy = (TPad*) plotPad->FindObject(padName); 01112 if(!paddy) 01113 errors++; 01114 } 01115 if(!errors) 01116 return; 01117 } 01118 01119 elecPadsDone=1; 01120 01121 01122 Double_t left[5]={0.04,0.23,0.42,0.61,0.80}; 01123 Double_t right[5]={0.23,0.42,0.61,0.80,0.99}; 01124 Double_t top[6]={0.93,0.78,0.63,0.48,0.33,0.18}; 01125 Double_t bottom[6]={0.78,0.63,0.48,0.33,0.18,0.03}; 01126 01127 //Now add some labels around the plot 01128 TLatex texy; 01129 texy.SetTextSize(0.03); 01130 texy.SetTextAlign(12); 01131 for(int column=0;column<5;column++) { 01132 sprintf(textLabel,"Chan %d/%d",1+(2*column),2+(2*column)); 01133 if(column==4) 01134 texy.DrawTextNDC(right[column]-0.1,0.97,textLabel); 01135 else 01136 texy.DrawTextNDC(right[column]-0.09,0.97,textLabel); 01137 } 01138 texy.SetTextAlign(21); 01139 texy.SetTextAngle(90); 01140 texy.DrawTextNDC(left[0]-0.01,bottom[0],"A"); 01141 texy.DrawTextNDC(left[0]-0.01,bottom[2],"B"); 01142 texy.DrawTextNDC(left[0]-0.01,bottom[4],"C"); 01143 01144 // A 01145 // 1 3 5 7 9 01146 // 2 4 6 8 01147 // B 01148 // 1 3 5 7 9 01149 // 2 4 6 8 01150 // C 01151 // 1 3 5 7 9 01152 // 2 4 6 8 01153 01154 int count=0; 01155 01156 01157 01158 01159 for(int row=0;row<6;row++) { 01160 for(int column=0;column<5;column++) { 01161 plotPad->cd(); 01162 // if(row%2==1 && column==4) continue; 01163 sprintf(padName,"elecChanPad%d",column+5*row); 01164 TPad *paddy1 = new TPad(padName,padName,left[column],bottom[row],right[column],top[row]); 01165 paddy1->SetTopMargin(0); 01166 paddy1->SetBottomMargin(0); 01167 paddy1->SetLeftMargin(0); 01168 paddy1->SetRightMargin(0); 01169 if(column==4) 01170 paddy1->SetRightMargin(0.01); 01171 if(column==0) 01172 paddy1->SetLeftMargin(0.1); 01173 if(row==5) 01174 paddy1->SetBottomMargin(0.1); 01175 paddy1->Draw(); 01176 paddy1->cd(); 01177 01178 TH1F *framey=0; 01179 01180 01181 // std::cout << "Limits\t" << fMinVoltLimit << "\t" << fMaxVoltLimit << "\n"; 01182 01183 01184 if(fWaveformOption==AraDisplayFormatOption::kWaveform || fWaveformOption==AraDisplayFormatOption::kHilbertEnvelope) 01185 framey = (TH1F*) paddy1->DrawFrame(0,fMinVoltLimit,250,fMaxVoltLimit); 01186 // framey = (TH1F*) paddy1->DrawFrame(fMinTimeLimit,fMinVoltLimit,fMaxTimeLimit,fMaxVoltLimit); 01187 else if(fWaveformOption==AraDisplayFormatOption::kFFT || fWaveformOption==AraDisplayFormatOption::kAveragedFFT) 01188 framey = (TH1F*) paddy1->DrawFrame(fMinFreqLimit,fMinPowerLimit,fMaxFreqLimit,fMaxPowerLimit); 01189 01190 framey->GetYaxis()->SetLabelSize(0.08); 01191 framey->GetYaxis()->SetTitleSize(0.1); 01192 framey->GetYaxis()->SetTitleOffset(0.5); 01193 01194 if(row==4) { 01195 framey->GetXaxis()->SetLabelSize(0.09); 01196 framey->GetXaxis()->SetTitleSize(0.09); 01197 framey->GetYaxis()->SetLabelSize(0.09); 01198 framey->GetYaxis()->SetTitleSize(0.09); 01199 } 01200 if(fWebPlotterMode && column!=0) { 01201 framey->GetYaxis()->SetLabelSize(0); 01202 framey->GetYaxis()->SetTitleSize(0); 01203 framey->GetYaxis()->SetTitleOffset(0); 01204 } 01205 count++; 01206 } 01207 } 01208 01209 } 01210 01211 01212 01213 void AraTBCanvasMaker::setupRFChanPadWithFrames(TPad *plotPad, Int_t stationId) 01214 { 01215 int maxColumns=0; 01216 int maxRows=0; 01217 int numRFChans=0; 01218 Double_t left[4]={0.04,0.27,0.50,0.73}; 01219 Double_t right[4]={0.27,0.50,0.73,0.96}; 01220 Double_t top[5]={0}; 01221 Double_t bottom[5]={0}; 01222 if(stationId==0){ 01223 maxColumns=4; 01224 maxRows=4; 01225 numRFChans=maxRows*maxColumns; 01226 01227 top[0]=0.95; 01228 top[1]=0.72; 01229 top[2]=0.49; 01230 top[3]=0.26; 01231 bottom[0]=0.72; 01232 bottom[1]=0.49; 01233 bottom[2]=0.26; 01234 bottom[3]=0.03; 01235 } 01236 01237 if(stationId==1){ 01238 maxColumns=4; 01239 maxRows=5; 01240 numRFChans=maxRows*maxColumns; 01241 01242 top[0]=0.95; 01243 top[1]=0.77; 01244 top[2]=0.59; 01245 top[3]=0.41; 01246 top[4]=0.23; 01247 bottom[0]=0.77; 01248 bottom[1]=0.59; 01249 bottom[2]=0.41; 01250 bottom[3]=0.23; 01251 bottom[4]=0.05; 01252 } 01253 01254 static int rfChanPadsDone=0; 01255 char textLabel[180]; 01256 char padName[180]; 01257 plotPad->cd(); 01258 if(fLastCanvasView!=AraDisplayCanvasLayoutOption::kRFChanView) { 01259 plotPad->Clear(); 01260 } 01261 01262 if(fRedoEventCanvas && rfChanPadsDone){ 01263 plotPad->Clear(); 01264 } 01265 01266 fLastCanvasView=AraDisplayCanvasLayoutOption::kRFChanView; 01267 01268 if(rfChanPadsDone && !fRedoEventCanvas) { 01269 int errors=0; 01270 for(int rfChan=0;rfChan<numRFChans;rfChan++) { 01271 sprintf(padName,"rfChanPad%d",rfChan); 01272 TPad *paddy = (TPad*) plotPad->FindObject(padName); 01273 if(!paddy) 01274 errors++; 01275 } 01276 if(!errors) 01277 return; 01278 } 01279 01280 01281 rfChanPadsDone=1; 01282 01283 01284 01285 01286 //Now add some labels around the plot 01287 TLatex texy; 01288 texy.SetTextSize(0.03); 01289 texy.SetTextAlign(12); 01290 for(int column=0;column<maxColumns;column++) { 01291 sprintf(textLabel,"%d/%d",1+column,5+column); 01292 if(column==3) 01293 texy.DrawTextNDC(right[column]-0.12,0.97,textLabel); 01294 else 01295 texy.DrawTextNDC(right[column]-0.12,0.97,textLabel); 01296 } 01297 texy.SetTextAlign(21); 01298 texy.SetTextAngle(90); 01299 texy.DrawTextNDC(left[0]-0.01,bottom[0]+0.1,"1-4"); 01300 texy.DrawTextNDC(left[0]-0.01,bottom[1]+0.1,"5-8"); 01301 texy.DrawTextNDC(left[0]-0.01,bottom[2]+0.1,"9-12"); 01302 texy.DrawTextNDC(left[0]-0.01,bottom[3]+0.1,"13-16"); 01303 if(stationId==1) texy.DrawTextNDC(left[0]-0.01,bottom[4]+0.1,"17-20"); 01304 01305 01306 01307 int count=0; 01308 01309 // 1 2 3 4 01310 // 5 6 7 8 01311 // 9 10 11 12 01312 // 13 14 15 16 01313 01314 for(int column=0;column<maxColumns;column++) { 01315 for(int row=0;row<maxRows;row++) { 01316 plotPad->cd(); 01317 // int rfChan=column+4*row; 01318 sprintf(padName,"rfChanPad%d",column+4*row); 01319 TPad *paddy1 = new TPad(padName,padName,left[column],bottom[row],right[column],top[row]); 01320 paddy1->SetTopMargin(0); 01321 paddy1->SetBottomMargin(0); 01322 paddy1->SetLeftMargin(0); 01323 paddy1->SetRightMargin(0); 01324 if(column==3) 01325 paddy1->SetRightMargin(0.01); 01326 if(column==0) 01327 paddy1->SetLeftMargin(0.1); 01328 if(row==3&&stationId==0) 01329 paddy1->SetBottomMargin(0.1); 01330 if(row==4&&stationId==1) 01331 paddy1->SetBottomMargin(0.1); 01332 paddy1->Draw(); 01333 paddy1->cd(); 01334 TH1F *framey=0; 01335 if(fWaveformOption==AraDisplayFormatOption::kFFT || fWaveformOption==AraDisplayFormatOption::kAveragedFFT){ 01336 framey = (TH1F*) paddy1->DrawFrame(fMinFreqLimit,fMinPowerLimit,fMaxFreqLimit,fMaxPowerLimit); 01337 } 01338 else if(fWaveformOption==AraDisplayFormatOption::kWaveform || 01339 fWaveformOption==AraDisplayFormatOption::kHilbertEnvelope) { 01340 if(row<3) { 01341 framey = (TH1F*) paddy1->DrawFrame(fMinTimeLimit,fMinVoltLimit,fMaxTimeLimit,fMaxVoltLimit); 01342 } 01343 else{ 01344 framey = (TH1F*) paddy1->DrawFrame(fMinTimeLimit,fMinClockVoltLimit,fMaxTimeLimit,fMaxClockVoltLimit); 01345 } 01346 } 01347 01348 framey->GetYaxis()->SetLabelSize(0.1); 01349 framey->GetYaxis()->SetTitleSize(0.1); 01350 framey->GetYaxis()->SetTitleOffset(0.5); 01351 if(row==4&&stationId==1) { 01352 framey->GetXaxis()->SetLabelSize(0.09); 01353 framey->GetXaxis()->SetTitleSize(0.09); 01354 framey->GetYaxis()->SetLabelSize(0.09); 01355 framey->GetYaxis()->SetTitleSize(0.09); 01356 } 01357 if(row==3&&stationId==0) { 01358 framey->GetXaxis()->SetLabelSize(0.09); 01359 framey->GetXaxis()->SetTitleSize(0.09); 01360 framey->GetYaxis()->SetLabelSize(0.09); 01361 framey->GetYaxis()->SetTitleSize(0.09); 01362 } 01363 01364 if(fWebPlotterMode && column!=0) { 01365 framey->GetYaxis()->SetLabelSize(0); 01366 framey->GetYaxis()->SetTitleSize(0); 01367 framey->GetYaxis()->SetTitleOffset(0); 01368 } 01369 01370 count++; 01371 } 01372 } 01373 } 01374 01375 01376 01377 void AraTBCanvasMaker::setupAntPadWithFrames(TPad *plotPad, Int_t stationId) 01378 { 01379 static int antPadsDone=0; 01380 char textLabel[180]; 01381 char padName[180]; 01382 plotPad->cd(); 01383 if(fLastCanvasView!=AraDisplayCanvasLayoutOption::kAntennaView) { 01384 plotPad->Clear(); 01385 } 01386 01387 if(fRedoEventCanvas && antPadsDone){ 01388 plotPad->Clear(); 01389 } 01390 01391 fLastCanvasView=AraDisplayCanvasLayoutOption::kAntennaView; 01392 01393 if(antPadsDone && !fRedoEventCanvas) { 01394 int errors=0; 01395 for(int column=0;column<4;column++) { 01396 for(int row=0;row<4;row++) { 01397 sprintf(padName,"antPad%d_%d",column,row); 01398 TPad *paddy = (TPad*) plotPad->FindObject(padName); 01399 if(!paddy) 01400 errors++; 01401 } 01402 } 01403 if(!errors) 01404 return; 01405 } 01406 01407 01408 antPadsDone=1; 01409 01410 01411 Double_t left[4]={0.04,0.27,0.50,0.73}; 01412 Double_t right[4]={0.27,0.50,0.73,0.96}; 01413 Double_t top[4]={0.95,0.72,0.49,0.26}; 01414 Double_t bottom[4]={0.72,0.49,0.26,0.03}; 01415 01416 //Now add some labels around the plot 01417 TLatex texy; 01418 texy.SetTextSize(0.03); 01419 texy.SetTextAlign(12); 01420 for(int column=0;column<4;column++) { 01421 sprintf(textLabel,"%d/%d",1+column,5+column); 01422 if(column==3) 01423 texy.DrawTextNDC(right[column]-0.12,0.97,textLabel); 01424 else 01425 texy.DrawTextNDC(right[column]-0.12,0.97,textLabel); 01426 } 01427 texy.SetTextAlign(21); 01428 texy.SetTextAngle(90); 01429 texy.DrawTextNDC(left[0]-0.01,bottom[0]+0.1,"V"); 01430 texy.DrawTextNDC(left[0]-0.01,bottom[1]+0.1,"V/S"); 01431 texy.DrawTextNDC(left[0]-0.01,bottom[2]+0.1,"H"); 01432 texy.DrawTextNDC(left[0]-0.01,bottom[3]+0.1,"H"); 01433 01434 01435 int count=0; 01436 01437 01438 01439 01440 for(int column=0;column<4;column++) { 01441 for(int row=0;row<4;row++) { 01442 plotPad->cd(); 01443 sprintf(padName,"antPad%d_%d",column,row); 01444 01445 TPad *paddy1 = new TPad(padName,padName,left[column],bottom[row],right[column],top[row]); 01446 paddy1->SetTopMargin(0); 01447 paddy1->SetBottomMargin(0); 01448 paddy1->SetLeftMargin(0); 01449 paddy1->SetRightMargin(0); 01450 if(column==3) 01451 paddy1->SetRightMargin(0.01); 01452 if(column==0) 01453 paddy1->SetLeftMargin(0.1); 01454 if(row==3) 01455 paddy1->SetBottomMargin(0.1); 01456 paddy1->Draw(); 01457 paddy1->cd(); 01458 TH1F *framey=0; 01459 if(fWaveformOption==AraDisplayFormatOption::kFFT || fWaveformOption==AraDisplayFormatOption::kAveragedFFT){ 01460 framey = (TH1F*) paddy1->DrawFrame(fMinFreqLimit,fMinPowerLimit,fMaxFreqLimit,fMaxPowerLimit); 01461 } 01462 else if(fWaveformOption==AraDisplayFormatOption::kWaveform || 01463 fWaveformOption==AraDisplayFormatOption::kHilbertEnvelope) { 01464 if(row<3) { 01465 framey = (TH1F*) paddy1->DrawFrame(fMinTimeLimit,fMinVoltLimit,fMaxTimeLimit,fMaxVoltLimit); 01466 } 01467 else{ 01468 framey = (TH1F*) paddy1->DrawFrame(fMinTimeLimit,fMinClockVoltLimit,fMaxTimeLimit,fMaxClockVoltLimit); 01469 } 01470 } 01471 01472 framey->GetYaxis()->SetLabelSize(0.1); 01473 framey->GetYaxis()->SetTitleSize(0.1); 01474 framey->GetYaxis()->SetTitleOffset(0.5); 01475 if(row==3) { 01476 framey->GetXaxis()->SetLabelSize(0.09); 01477 framey->GetXaxis()->SetTitleSize(0.09); 01478 framey->GetYaxis()->SetLabelSize(0.09); 01479 framey->GetYaxis()->SetTitleSize(0.09); 01480 } 01481 if(fWebPlotterMode && column!=0) { 01482 framey->GetYaxis()->SetLabelSize(0); 01483 framey->GetYaxis()->SetTitleSize(0); 01484 framey->GetYaxis()->SetTitleOffset(0); 01485 } 01486 count++; 01487 } 01488 } 01489 } 01490 01491 01492 01493 void AraTBCanvasMaker::deleteTGraphsFromElecPad(TPad *paddy,int chan) 01494 { 01495 paddy->cd(); 01496 if(fLastWaveformFormat==AraDisplayFormatOption::kWaveform) paddy->GetListOfPrimitives()->Remove(grTBElec[chan]); 01497 else if(fLastWaveformFormat==AraDisplayFormatOption::kFFT) paddy->GetListOfPrimitives()->Remove(grTBElecFFT[chan]); 01498 else if(fLastWaveformFormat==AraDisplayFormatOption::kAveragedFFT) paddy->GetListOfPrimitives()->Remove(grTBElecAveragedFFT[chan]); 01499 else if(fLastWaveformFormat==AraDisplayFormatOption::kHilbertEnvelope) paddy->GetListOfPrimitives()->Remove(grTBElecHilbert[chan]); 01500 // paddy->Update(); 01501 } 01502 01503 01504 void AraTBCanvasMaker::deleteTGraphsFromRFPad(TPad *paddy,int rfchan) 01505 { 01506 paddy->cd(); 01507 if(fLastWaveformFormat==AraDisplayFormatOption::kWaveform) paddy->GetListOfPrimitives()->Remove(grTBRFChan[rfchan]); 01508 else if(fLastWaveformFormat==AraDisplayFormatOption::kFFT) paddy->GetListOfPrimitives()->Remove(grTBRFChanFFT[rfchan]); 01509 else if(fLastWaveformFormat==AraDisplayFormatOption::kAveragedFFT) paddy->GetListOfPrimitives()->Remove(grTBRFChanAveragedFFT[rfchan]); 01510 else if(fLastWaveformFormat==AraDisplayFormatOption::kHilbertEnvelope) paddy->GetListOfPrimitives()->Remove(grTBRFChanHilbert[rfchan]); 01511 // paddy->Update(); 01512 } 01513 01514 01515 void AraTBCanvasMaker::resetAverage() 01516 { 01517 for(int chan=0;chan<NUM_DIGITIZED_ICRR_CHANNELS;chan++) { 01518 if(grTBElecAveragedFFT[chan]) { 01519 delete grTBElecAveragedFFT[chan]; 01520 grTBElecAveragedFFT[chan]=0; 01521 } 01522 } 01523 for(int rfchan=0;rfchan<RFCHANS_PER_ICRR;rfchan++) { 01524 if(grTBRFChanAveragedFFT[rfchan]) { 01525 delete grTBRFChanAveragedFFT[rfchan]; 01526 grTBRFChanAveragedFFT[rfchan]=0; 01527 } 01528 } 01529 } 01530 01531 01532
Generated on Wed Aug 8 16:20:07 2012 for ARA ROOT Test Bed Software by
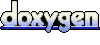