calibration/ICRR/TestBed/doInterleaveCal.cxx
00001 #include <iostream> 00002 #include <fstream> 00003 00004 //Event Reader Includes 00005 #include "UsefulAraTestBedStationEvent.h" 00006 #include "RawAraTestBedStationEvent.h" 00007 #include "AraGeomTool.h" 00008 #include "AraEventCalibrator.h" 00009 #include "araTestbedDefines.h" 00010 00011 //ROOT Includes 00012 #include "TROOT.h" 00013 #include "TCanvas.h" 00014 #include "TTree.h" 00015 #include "TFile.h" 00016 #include "TH1.h" 00017 #include "TGraphErrors.h" 00018 #include "TF1.h" 00019 #include "TTree.h" 00020 #include "TTreeIndex.h" 00021 #include "TButton.h" 00022 #include "TGroupButton.h" 00023 #include "TThread.h" 00024 #include "TEventList.h" 00025 #include "TMath.h" 00026 #include "TAxis.h" 00027 #include <TGClient.h> 00028 00029 #include <zlib.h> 00030 00031 00032 Double_t estimateLag(TGraph *grIn, Double_t freq); 00033 Double_t estimateLagLast(TGraph *grIn, Double_t freq); 00034 Double_t mySineWave(Double_t *t, Double_t *par) ; 00035 int gotPedFile=0; 00036 char pedFile[FILENAME_MAX]; 00037 float pedestalData[LAB3_PER_TESTBED][CHANNELS_PER_LAB3][MAX_NUMBER_SAMPLES_LAB3]; 00038 Double_t binWidths[LAB3_PER_TESTBED][2][MAX_NUMBER_SAMPLES_LAB3]; 00039 00040 int main(int argc, char **argv) 00041 { 00042 char inputFile[FILENAME_MAX]; 00043 Double_t ampVal=200; 00044 Double_t freqVal=0.2; //200 Mhz 00045 00046 if(argc>1) { 00047 strncpy(inputFile,argv[1],FILENAME_MAX); 00048 } 00049 else { 00050 strncpy(inputFile,"/unix/anita1/ara/data/frozen_daqbox_calibration/Minus54C/sine_wave_data/root/event250MHz_331mV.root",FILENAME_MAX); 00051 } 00052 if(argc>2) { 00053 freqVal=atof(argv[2]); 00054 } 00055 if(argc>3) { 00056 ampVal=atof(argv[3]); 00057 } 00058 00059 00060 // TFile *fp = new TFile("/Users/rjn/ara/data/root/event_frozen_200MHz.root"); 00061 TFile *fp = new TFile(inputFile); 00062 if(!fp) { 00063 std::cerr << "Can't open file\n"; 00064 return -1; 00065 } 00066 TTree *eventTree = (TTree*) fp->Get("eventTree"); 00067 if(!eventTree) { 00068 std::cerr << "Can't find eventTree\n"; 00069 return -1; 00070 } 00071 // AraEventCalibrator::Instance()->setPedFile("/Users/rjn/ara/data/frozen_daqbox_calibration/Minus54C/pedestal_files/peds_1291239657/peds_1291239657/peds_1291239657.193855.dat"); 00072 AraEventCalibrator::Instance()->setPedFile("/unix/anita1/ara/data/frozen_daqbox_calibration/Minus54C/pedestal_files/peds_1291239657/peds_1291239657/peds_1291239657.193855.dat"); 00073 RawAraTestBedStationEvent *evPtr=0; 00074 eventTree->SetBranchAddress("event",&evPtr); 00075 00076 Double_t period=1./freqVal; 00077 00078 00079 char outName[180]; 00080 sprintf(outName,"interleaveFile_%3.0f_%3.0f.root",1000*freqVal,ampVal); 00081 TFile *fpInterleave = new TFile(outName,"RECREATE"); 00082 TH1F *histInterleave[2]; 00083 TH1F *histInterleaveChan[2][4]; 00084 char histName[180]; 00085 00086 for(int chip=0;chip<2;chip++) { 00087 sprintf(histName,"histInterleave_%d",chip); 00088 histInterleave[chip] = new TH1F(histName,histName,10000,-period,period); 00089 for(int chan=0;chan<4;chan++) { 00090 sprintf(histName,"histInterleaveChan_%d_%d",chip,chan); 00091 histInterleaveChan[chip][chan] = new TH1F(histName,histName,10000,-period,period); 00092 } 00093 00094 } 00095 AraGeomTool *tempGeom = AraGeomTool::Instance(); 00096 00097 Long64_t numEntries=eventTree->GetEntries(); 00098 Long64_t starEvery=numEntries/80; 00099 if(starEvery==0) starEvery++; 00100 for(Long64_t i=0;i<numEntries;i++) { 00101 00102 00103 if(i%starEvery==0) { 00104 std::cerr << "*"; 00105 00106 std::ofstream EvNum("lastEvent"); 00107 EvNum << i << "\n"; 00108 EvNum.close(); 00109 } 00110 eventTree->GetEntry(i); 00111 // std::cerr << i << "\t" << evPtr->head.unixTime << "\n"; 00112 // for(int chan=0;chan<NUM_DIGITIZED_TESTBED_CHANNELS;chan++) { 00113 if(evPtr->getFirstHitBus(18)!=evPtr->getFirstHitBus(19)) { 00114 std::cerr << "Bad channel?\n"; 00115 continue; 00116 } 00117 if(evPtr->head.unixTime>2e9) continue; 00118 00119 00120 UsefulAraTestBedStationEvent realEvent(evPtr,AraCalType::kFirstCalib); 00121 00122 for(int rfChan=0;rfChan<8;rfChan++) { 00123 int ci1=tempGeom->getFirstLabChanIndexForChan(rfChan); 00124 int ci2=tempGeom->getSecondLabChanIndexForChan(rfChan); 00125 int chip=ci1/9; 00126 00127 Int_t firstSamp=evPtr->getEarliestSample(ci1); 00128 if(firstSamp<20 || firstSamp>250) continue; 00129 00130 TGraph *grFirst = realEvent.getGraphFromElecChan(ci1); 00131 TGraph *grSecond = realEvent.getGraphFromElecChan(ci2); 00132 Double_t lag1=estimateLag(grFirst,freqVal); 00133 Double_t lag2=estimateLag(grSecond,freqVal); 00134 Double_t shift=lag1-lag2; 00135 if(TMath::Abs(shift+period)<TMath::Abs(shift)) shift+=period; 00136 if(TMath::Abs(shift-period)<TMath::Abs(shift)) shift-=period; 00137 histInterleave[chip]->Fill(shift); 00138 histInterleaveChan[chip][rfChan%4]->Fill(shift); 00139 // Double_t *xVals = grSecond->GetX(); 00140 // Double_t *yVals = grSecond->GetY(); 00141 // Int_t numVals=grSecond->GetN(); 00142 // for(int i=0;i<numVals;i++) { 00143 // xVals[i]+=shift; 00144 // } 00145 // TGraph *grSecondCor = new TGraph(numVals,xVals,yVals); 00146 // std::cout << lag1 << "\t" << lag2 << "\n"; 00147 // TCanvas *can = new TCanvas(); 00148 // grFirst->Draw("alp"); 00149 // grSecondCor->SetMarkerColor(8); 00150 // grSecondCor->SetLineColor(8); 00151 // grSecondCor->Draw("lp"); 00152 delete grFirst; 00153 delete grSecond; 00154 } 00155 } 00156 00157 fpInterleave->Write(); 00158 sprintf(outName,"interleaveFile_%3.0f_%3.0f.txt",1000*freqVal,ampVal); 00159 std::ofstream IntFile(outName); 00160 for(int chip=0;chip<2;chip++) { 00161 for (int chan=0;chan<4;chan++) { 00162 histInterleaveChan[chip][chan]->GetXaxis()->SetRangeUser(-1,1); 00163 IntFile << chip << "\t" << chan << "\t" << histInterleaveChan[chip][chan]->GetMean() << "\n"; 00164 } 00165 } 00166 IntFile.close(); 00167 00168 } 00169 00170 00171 00172 void loadPedestals() 00173 { 00174 if(!gotPedFile) { 00175 char calibDir[FILENAME_MAX]; 00176 char *calibEnv=getenv("ARA_CALIB_DIR"); 00177 if(!calibEnv) { 00178 char *utilEnv=getenv("ARA_UTIL_INSTALL_DIR"); 00179 if(!utilEnv) 00180 sprintf(calibDir,"calib"); 00181 else 00182 sprintf(calibDir,"%s/share/araCalib",utilEnv); 00183 } 00184 else { 00185 strncpy(calibDir,calibEnv,FILENAME_MAX); 00186 } 00187 sprintf(pedFile,"%s/peds_1286989711.394723.dat",calibDir); 00188 } 00189 FullLabChipPedStruct_t peds; 00190 gzFile inPed = gzopen(pedFile,"r"); 00191 if( !inPed ){ 00192 fprintf(stderr,"Failed to open pedestal file %s.\n",pedFile); 00193 return; 00194 } 00195 00196 int nRead = gzread(inPed,&peds,sizeof(FullLabChipPedStruct_t)); 00197 if( nRead != sizeof(FullLabChipPedStruct_t)){ 00198 int numErr; 00199 fprintf(stderr,"Error reading pedestal file %s; %s\n",pedFile,gzerror(inPed,&numErr)); 00200 gzclose(inPed); 00201 return; 00202 } 00203 00204 int chip,chan,samp; 00205 for(chip=0;chip<LAB3_PER_TESTBED;++chip) { 00206 for(chan=0;chan<CHANNELS_PER_LAB3;++chan) { 00207 int chanIndex = chip*CHANNELS_PER_LAB3+chan; 00208 for(samp=0;samp<MAX_NUMBER_SAMPLES_LAB3;++samp) { 00209 pedestalData[chip][chan][samp]=peds.chan[chanIndex].pedMean[samp]; 00210 } 00211 } 00212 } 00213 gzclose(inPed); 00214 } 00215 00216 00217 00218 00219 Double_t mySineWave(Double_t *t, Double_t *par) 00220 { 00221 Double_t amp=par[0]; //In mV 00222 Double_t freq=par[1]; //In GHz 00223 Double_t lag=par[2]; //In ns 00224 00225 return amp*TMath::Sin(TMath::TwoPi()*freq*(t[0]-lag)); 00226 00227 } 00228 00229 void loadCalib() 00230 { 00231 std::ifstream BinFile("binWidths.txt"); 00232 int chip,rco; 00233 double width; 00234 while(BinFile >> chip >> rco) { 00235 for(int samp=0;samp<MAX_NUMBER_SAMPLES_LAB3;samp++) { 00236 BinFile >> width; 00237 binWidths[chip][rco][samp]=width; 00238 } 00239 } 00240 } 00241 00242 Double_t estimateLag(TGraph *grIn, Double_t freq) 00243 { 00244 // This funciton estimates the lag by just using all the negative-positive zero crossing 00245 00246 Int_t numPoints=grIn->GetN(); 00247 if(numPoints<3) return 0; 00248 Double_t period=1./freq; 00249 Double_t *tVals=grIn->GetX(); 00250 Double_t *vVals=grIn->GetY(); 00251 00252 Double_t zc[1000]={0}; 00253 Double_t rawZc[1000]={0}; 00254 int countZC=0; 00255 for(int i=2;i<numPoints;i++) { 00256 if(vVals[i-1]<0 && vVals[i]>0) { 00257 Double_t x1=tVals[i-1]; 00258 Double_t x2=tVals[i]; 00259 Double_t y1=vVals[i-1]; 00260 Double_t y2=vVals[i]; 00261 // std::cout << i << "\t" << y2 << "\t" << y1 << "\t" << (y2-y1) << "\n"; 00262 zc[countZC]=(((0-y1)/(y2-y1))*(x2-x1))+x1; 00263 rawZc[countZC]=zc[countZC]; 00264 countZC++; 00265 // if(countZC==1) 00266 // break; 00267 } 00268 } 00269 00270 Double_t firstZC=zc[0]; 00271 while(firstZC>period) firstZC-=period; 00272 Double_t meanZC=0; 00273 for(int i=0;i<countZC;i++) { 00274 while((zc[i]-firstZC)>period) zc[i]-=period; 00275 if(TMath::Abs((zc[i]-period)-firstZC)<TMath::Abs(zc[i]-firstZC)) 00276 zc[i]-=period; 00277 meanZC+=zc[i]; 00278 // std::cout << i << "\t" << zc[i] << "\n"; 00279 } 00280 // TCanvas *can = new TCanvas(); 00281 // TGraph *gr = new TGraph(countZC,rawZc,zc); 00282 // gr->Draw("ap"); 00283 00284 // std::cout << "\n"; 00285 meanZC/=countZC; 00286 00287 // std::cout << zc << "\n"; 00288 return meanZC; 00289 00290 } 00291 00292 00293 00294 Double_t estimateLagLast(TGraph *grIn, Double_t freq) 00295 { 00296 00297 // This funciton estimates the lag by just using the first negative-positive zero crossing 00298 // To resolve quadrant ambiguity in the ASin function, the first zero crossing is used as a test of lag 00299 Int_t numPoints=grIn->GetN(); 00300 if(numPoints<3) return 0; 00301 Double_t period=1/freq; 00302 Double_t *tVals=grIn->GetX(); 00303 Double_t *vVals=grIn->GetY(); 00304 00305 Double_t zc=0; 00306 for(int i=2;i<numPoints;i++) { 00307 if(vVals[i-1]<0 && vVals[i]>0) { 00308 Double_t x1=tVals[i-1]; 00309 Double_t x2=tVals[i]; 00310 Double_t y1=vVals[i-1]; 00311 Double_t y2=vVals[i]; 00312 // std::cout << i << "\t" << y2 << "\t" << y1 << "\t" << (y2-y1) << "\n"; 00313 zc=(((0-y1)/(y2-y1))*(x2-x1))+x1; 00314 // break; 00315 } 00316 } 00317 while(zc>period) zc-=period; 00318 // std::cout << zc << "\n"; 00319 return zc; 00320 00321 }
Generated on Wed Aug 8 16:20:07 2012 for ARA ROOT Test Bed Software by
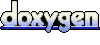