AraWebPlotter/kvpLib/keyValuePair.h
00001 /* keyValuePair.h - <short description of this file/module> */ 00002 00003 /* G.J.Crone, University College London */ 00004 00005 /* 00006 * Current CVS Tag: 00007 * $Header: /work1/anitaCVS/flightSoft/common/kvpLib/keyValuePair.h,v 1.1 2004/08/31 15:48:09 rjn Exp $ 00008 */ 00009 00010 /* 00011 * Modification History : DO NOT EDIT - MAINTAINED BY CVS 00012 * $Log: keyValuePair.h,v $ 00013 * Revision 1.1 2004/08/31 15:48:09 rjn 00014 * Um... lots of additions. The biggest ones are switching from shared to static libraries, and the additon of configLib and kvpLib to read formatted config files. These two libraries were created by Gordon Crone (gjc@hep.ucl.ac.uk) and are in spirit released under the GPL license. 00015 * Also the first of the actually programs (Eventd) has been started. At the moment it just opens a socket to fakeAcqd. Other sockets and better handling of multiple sockets in socketLib are the next things on my to do list. 00016 * 00017 * Revision 1.11 2004/03/04 16:15:56 gjc 00018 * Grow internal buffer (by allocating larger buffer and copying data) as 00019 * necessary. 00020 * 00021 * Revision 1.10 2004/03/04 11:50:20 gjc 00022 * Added new function kvpQueryLength to return the number of elements in 00023 * an array. 00024 * 00025 * Revision 1.9 2004/01/23 17:40:24 gjc 00026 * double size of buffer (KVP_BUFFER_SIZE). 00027 * 00028 * Revision 1.8 2003/09/04 21:06:16 gjc 00029 * Use malloc instead of static array. Move BUFFER_SIZE in keyValuePair.c to KVP_BUFFER_SIZE in keyValuePair.h 00030 * 00031 * Revision 1.7 2003/07/31 16:53:01 gjc 00032 * Invalidate old key and skip it from kvpFormat when overwriting in kvpParse 00033 * 00034 * Revision 1.6 2003/02/27 12:34:36 gjc 00035 * Robert Hatcher's mods to avoid warnings with gcc 3.2 00036 * 00037 * Revision 1.1 2003/02/25 21:18:40 rhatcher 00038 * key-value pair string en/decoding that the DAQ group uses. Comes through 00039 * in the RawRunConfigBlock (which holds the "run prepare" string). 00040 * small modifications to "official" version to eliminate warning messages. 00041 * 00042 * Revision 1.5 2003/02/13 15:41:18 gjc 00043 * Increased MAXKEYS and check for exceding it in kvpParse 00044 * 00045 * Revision 1.4 2001/07/24 17:19:55 gjc 00046 * Added new function kvpGetStringArray 00047 * 00048 * Revision 1.3 2001/05/16 11:52:09 gjc 00049 * Added new function kvpUpdateInt needed for run number kludge. 00050 * Removed reference to syslog and added new error code KVP_E_TERMINATOR 00051 * for missing semi-colon. 00052 * 00053 * Revision 1.2 2001/04/11 16:27:05 gjc 00054 * Stricter checking of numeric values in kvpParse. Fixes bug with 00055 * inconsistent element count for hex values. 00056 * No longer start again at beginnning of internal buffers every time 00057 * kvpParse is a called. Must call kvpReset before kvpParse if that's 00058 * what you want. 00059 * 00060 * Revision 1.1 2001/03/26 18:09:21 gjc 00061 * initial check in 00062 * 00063 * 00064 */ 00065 00066 #ifndef _keyValuePair_H 00067 #define _keyValuePair_H 00068 #ifdef __cplusplus 00069 extern "C" { 00070 #endif /* __cplusplus */ 00071 00072 /* includes */ 00073 00074 /* defines */ 00075 #define MAXKEYS 1000 00076 #define KVP_MIN_BUFFER_SIZE 20480 00077 00078 #define MAX_INT_LENGTH 12 00079 #define MAX_FLOAT_LENGTH 14 00080 00081 /* typedefs */ 00082 typedef enum { 00083 KVP_E_OK = 0, 00084 KVP_E_DUPLICATE, 00085 KVP_E_NOTFOUND, 00086 KVP_E_SYSTEM, 00087 KVP_E_BADKEY, 00088 KVP_E_BADVALUE, 00089 KVP_E_TOOMANY, 00090 KVP_E_TOOLONG, 00091 KVP_E_BADTYPE, 00092 KVP_E_BADINPUT, 00093 KVP_E_WRONGCOUNT, 00094 KVP_E_TRUNCATE, 00095 KVP_E_ILLNUMBER, 00096 KVP_E_TERMINATOR 00097 } KvpErrorCode ; 00098 00099 typedef enum { 00100 KT_STRING, 00101 KT_FLOAT, 00102 KT_INT, 00103 KT_UINT, 00104 KT_INVALID 00105 } KeyType ; 00106 /* global variable declarations*/ 00107 00108 /* function prototype declarations */ 00109 void kvpInit (int bufferSize) ; 00110 int kvpParse (const char* record) ; 00111 00112 void kvpReset (void) ; 00113 KvpErrorCode kvpGetEntry (int entry, char** key, KeyType* type, 00114 int*size, char** value) ; 00115 00116 char* kvpGetString (const char* key) ; 00117 00118 int kvpGetInt (const char* key, int defaultValue) ; 00119 int kvpGetDec (const char* key, int defaultValue) ; 00120 int kvpGetHex (const char* key, int defaultValue) ; 00121 unsigned int kvpGetUnsignedInt (const char* key,unsigned int defaultValue); 00122 00123 00124 float kvpGetFloat (const char* key, float defaultValue) ; 00125 double kvpGetDouble (const char* key, double defaultValue) ; 00126 00127 int kvpQueryLength (const char* key) ; 00128 void kvpDumpInternals (void) ; 00129 00130 KvpErrorCode kvpSetString (const char* key, const char* value) ; 00131 KvpErrorCode kvpSetInt (const char* key, int value) ; 00132 KvpErrorCode kvpSetFloat (const char* key, float value) ; 00133 00134 KvpErrorCode kvpSetIntArray (const char* key, const int* values, 00135 int count) ; 00136 KvpErrorCode kvpSetFloatArray (const char* key, const float* values, 00137 int count) ; 00138 00139 KvpErrorCode kvpGetIntArray (const char* key, 00140 int* values, int* entries) ; 00141 KvpErrorCode kvpGetFloatArray (const char* key, 00142 float* values, int* entries) ; 00143 00144 KvpErrorCode kvpGetStringArray (const char* key, char* values, 00145 int maxlen, int* entries) ; 00146 00147 KvpErrorCode kvpUpdateInt (const char* key, int value) ; 00148 KvpErrorCode kvpUpdateIntArray (const char* key, int *values, 00149 int count) ; 00150 KvpErrorCode kvpUpdateUnsignedInt (const char* key, unsigned int value) ; 00151 00152 KvpErrorCode kvpUpdateUnsignedIntArray (const char* key, unsigned int *values, 00153 int count) ; 00154 KvpErrorCode kvpUpdateFloatArray (const char* key, float *values, 00155 int count) ; 00156 00157 KvpErrorCode kvpUpdateFloat (const char* key, float value) ; 00158 KvpErrorCode kvpUpdateString (const char* key,const char *value); 00159 00160 int kvpWrite (int stream) ; 00161 int kvpFormat (char* buffer, int length) ; 00162 00163 KvpErrorCode kvpError (void) ; 00164 void kvpPrintError (KvpErrorCode code) ; 00165 const char* kvpErrorString (KvpErrorCode code) ; 00166 #ifdef __cplusplus 00167 } 00168 #endif /* __cplusplus */ 00169 00170 #endif /* _keyValuePair_H */
Generated on Wed Aug 8 16:20:07 2012 for ARA ROOT Test Bed Software by
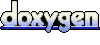