utilities/Icrr/makeAraHkTree.cxx
00001 #include <cstdio> 00002 #include <fstream> 00003 #include <iostream> 00004 #include <zlib.h> 00005 #include <libgen.h> 00006 #include <stdlib.h> 00007 00008 using namespace std; 00009 00010 #include "TTree.h" 00011 #include "TFile.h" 00012 #include "TSystem.h" 00013 00014 #define HACK_FOR_ROOT 00015 00016 #include "araIcrrStructures.h" 00017 #include "FullIcrrHkEvent.h" 00018 00019 void processHk(); 00020 void makeHkTree(char *inputName, char *outDir); 00021 00022 IcrrHkBody_t theHkBody; 00023 TFile *theFile; 00024 TTree *hkTree; 00025 FullIcrrHkEvent *theHk=0; 00026 char outName[FILENAME_MAX]; 00027 UInt_t realTime; 00028 Int_t runNumber; 00029 Int_t lastRunNumber; 00030 00031 00032 int main(int argc, char **argv) { 00033 if(argc<3) { 00034 std::cout << "Usage: " << basename(argv[0]) << " <file list> <out dir>" << std::endl; 00035 return -1; 00036 } 00037 if(argc==4) 00038 runNumber=atoi(argv[3]); 00039 makeHkTree(argv[1],argv[2]); 00040 return 0; 00041 } 00042 00043 00044 void makeHkTree(char *inputName, char *outFile) { 00045 cout << inputName << "\t" << outFile << endl; 00046 strncpy(outName,outFile,FILENAME_MAX); 00047 theHk = new FullIcrrHkEvent(); 00048 // cout << sizeof(IcrrHkBody_t) << endl; 00049 ifstream SillyFile(inputName); 00050 00051 int numBytes=0; 00052 char fileName[180]; 00053 int error=0; 00054 // int eventNumber=1; 00055 int counter=0; 00056 while(SillyFile >> fileName) { 00057 if(counter%100==0) 00058 cout << fileName << endl; 00059 counter++; 00060 00061 // const char *subDir = gSystem->DirName(fileName); 00062 // const char *subSubDir = gSystem->DirName(subDir); 00063 // const char *eventDir = gSystem->DirName(subSubDir); 00064 // const char *runDir = gSystem->DirName(eventDir); 00065 // const char *justRun = gSystem->BaseName(runDir); 00066 // cout << justRun << endl; 00067 // sscanf(justRun,"run%d",&runNumber); 00068 00069 gzFile infile = gzopen (fileName, "rb"); 00070 for(int i=0;i<1000;i++) { 00071 // cout << i << endl; 00072 numBytes=gzread(infile,&theHkBody,sizeof(IcrrHkBody_t)); 00073 if(numBytes!=sizeof(IcrrHkBody_t)) { 00074 if(numBytes) 00075 cerr << "Read problem: " <<numBytes << " of " << sizeof(IcrrHkBody_t) << endl; 00076 error=1; 00077 break; 00078 } 00079 // cout << "Hk: " << theHkBody.hd.eventNumber << endl; 00080 processHk(); 00081 } 00082 gzclose(infile); 00083 // if(error) break; 00084 } 00085 hkTree->AutoSave(); 00086 // theFile->Close(); 00087 } 00088 00089 00090 void processHk() { 00091 // cout << "processHk:\t" << theHkBody.eventNumber << endl; 00092 static int doneInit=0; 00093 00094 if(!doneInit) { 00095 // char dirName[FILENAME_MAX]; 00096 // char fileName[FILENAME_MAX]; 00097 // sprintf(dirName,"%s/run%d",outDirName,runNumber); 00098 // gSystem->mkdir(dirName,kTRUE); 00099 // sprintf(fileName,"%s/eventFile%d.root",dirName,runNumber); 00100 cout << "Creating File: " << outName << endl; 00101 theFile = new TFile(outName,"RECREATE"); 00102 hkTree = new TTree("hkTree","Tree of ARA Hks"); 00103 hkTree->Branch("run",&runNumber,"run/I"); 00104 hkTree->Branch("event","FullIcrrHkEvent",&theHk); 00105 00106 doneInit=1; 00107 } 00108 // cout << "Here: " << theHk.eventNumber << endl; 00109 if(theHk) delete theHk; 00110 theHk = new FullIcrrHkEvent(&theHkBody); 00111 hkTree->Fill(); 00112 lastRunNumber=runNumber; 00113 // delete theHk; 00114 }
Generated on Wed Aug 8 16:20:08 2012 for ARA ROOT Test Bed Software by
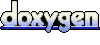