AraWebPlotter/AraTimeHistoHandler.cxx
00001 #include "AraTimeHistoHandler.h" 00002 #include <ctime> 00003 #include <iostream> 00004 00005 ClassImp(AraTimeHistoHandler); 00006 00007 AraTimeHistoHandler::AraTimeHistoHandler() 00008 { 00009 fBinWidthInSeconds=60; 00010 } 00011 00012 AraTimeHistoHandler::AraTimeHistoHandler( const char *name, const char *title , Int_t binWidth ):TNamed(name,title) 00013 { 00014 //Default constructor 00015 fBinWidthInSeconds=binWidth; 00016 } 00017 00018 void AraTimeHistoHandler::addAraTimeHistoHandler(AraTimeHistoHandler *other) 00019 { 00020 for(variableMap::iterator otherIt=other->theMap.begin(); 00021 otherIt!=other->theMap.end(); 00022 otherIt++) { 00023 UInt_t keyValue=(otherIt->first*other->fBinWidthInSeconds)/fBinWidthInSeconds; 00024 variableMap::iterator it=theMap.find(keyValue); 00025 variableMap::iterator itSq=theMapSq.find(keyValue); 00026 variableMap::iterator otherSqIt=other->theMapSq.find(otherIt->first); 00027 if(otherSqIt==other->theMapSq.end()) { 00028 std::cerr << "Consistency problem got variable but not variable squared\n"; 00029 continue; 00030 } 00031 if(it==theMap.end()) { 00032 theMap[keyValue]=otherIt->second; 00033 theMapSq[keyValue]=otherSqIt->second; 00034 } 00035 else { 00036 if(itSq==theMapSq.end()) { 00037 std::cerr << "Consistency problem got variable but not variable squared\n"; 00038 continue; 00039 } 00040 (it->second).first+=(otherIt->second).first; //This is the count 00041 (it->second).second+=(otherIt->second).second; //This is the variable 00042 (itSq->second).first+=(otherSqIt->second).first; //This is the count 00043 (itSq->second).second+=(otherSqIt->second).second; //This is the variable 00044 } 00045 } 00046 00047 } 00048 00049 00050 void AraTimeHistoHandler::addVariable(UInt_t unixTime, Double_t variable) 00051 { 00052 // std::cout << unixTime << "\t" << variable << "\n"; 00053 UInt_t keyValue=unixTime/fBinWidthInSeconds; 00054 variableMap::iterator it=theMap.find(keyValue); 00055 if(it==theMap.end()) { 00056 //This is the first entry 00057 variablePair first(1,variable); 00058 theMap[keyValue]=first; 00059 variablePair firstSq(1,variable*variable); 00060 theMapSq[keyValue]=firstSq; 00061 } 00062 else { 00063 theMap[keyValue].first++; 00064 theMap[keyValue].second+=variable; 00065 theMapSq[keyValue].first++; 00066 theMapSq[keyValue].second+=variable*variable; 00067 } 00068 } 00069 00070 TGraph *AraTimeHistoHandler::getTimeGraph(UInt_t firstTime, UInt_t lastTime, Int_t numPoints) 00071 { 00072 if(theMap.size()==0) return NULL; 00073 variableMap::iterator firstIt=theMap.lower_bound(firstTime/fBinWidthInSeconds); 00074 if(firstIt==theMap.end()) return NULL; 00075 variableMap::iterator lastIt=theMap.upper_bound(lastTime/fBinWidthInSeconds); 00076 if(lastIt==theMap.end()) lastIt--; //Decrement if we have already reached the end 00077 00078 UInt_t firstKey=firstIt->first; 00079 UInt_t lastKey=lastIt->first; 00080 UInt_t stepSize=(lastKey-firstKey)/(numPoints); 00081 // if(stepSize==0) 00082 stepSize++; 00083 // std::cout << "Keys: " << firstKey << "\t" << lastKey << "\t" << stepSize << "\n"; 00084 Int_t safetyFactor=10; 00085 Double_t *times = new Double_t[numPoints+safetyFactor]; 00086 Double_t *values = new Double_t[numPoints+safetyFactor]; 00087 Double_t *numEnts = new Double_t[numPoints+safetyFactor]; 00088 for(int i=0;i<numPoints+safetyFactor;i++) { 00089 values[i]=0; 00090 numEnts[i]=0; 00091 } 00092 lastIt++; //To include lastIt 00093 for(variableMap::iterator it=firstIt;it!=lastIt;it++) { 00094 // std::cout << (it->first) << "\t" << (it->second).second << "\n"; 00095 Int_t bin=(it->first-firstKey)/stepSize; 00096 // std::cout << "bin: " << bin << "\n"; 00097 values[bin]+=(it->second).second; 00098 numEnts[bin]+=(it->second).first; 00099 } 00100 00101 int counter=0; 00102 for(int i=0;i<numPoints;i++) { 00103 if(numEnts[i]>0) { 00104 times[counter]=fBinWidthInSeconds*(firstKey+i*stepSize); 00105 values[i]/=numEnts[i]; 00106 values[counter]=values[i]; 00107 counter++; 00108 } 00109 } 00110 //for(int i=0;i<counter;i++) { 00111 // std::cerr << "\t" << i << "\t" << numPoints << "\t" << times[i] << "\t" << values[i] << "\n"; 00112 // } 00113 00114 TGraph *gr=NULL; 00115 if(counter>1) 00116 gr = new TGraph(counter,times,values); 00117 // delete [] times; 00118 // delete [] values; 00119 // delete [] numEnts; 00120 return gr; 00121 00122 } 00123 00124 TGraph *AraTimeHistoHandler::getTimeGraph(AraPlotTime::AraPlotTime_t plotTime, Int_t numPoints) 00125 { 00126 00127 if(theMap.size()==0) return NULL; 00128 UInt_t lastTime=getLastTime(); 00129 // std::cout << this->GetName() << "\t" << plotTime << "\t" << getStartTime(lastTime,plotTime) << "\t" << lastTime << "\n"; 00130 return getTimeGraph(AraPlotTime::getStartTime(lastTime,plotTime),lastTime,numPoints); 00131 } 00132 00133 00134 TGraph *AraTimeHistoHandler::getCurrentTimeGraph(AraPlotTime::AraPlotTime_t plotTime, Int_t numPoints) 00135 { 00136 00137 if(theMap.size()==0) return NULL; 00138 UInt_t lastTime=time(NULL); 00139 return getTimeGraph(AraPlotTime::getStartTime(lastTime,plotTime),lastTime,numPoints); 00140 } 00141 00142 UInt_t AraTimeHistoHandler::getLastTime() 00143 { 00144 if(theMap.size()==0) return 0; 00145 variableMap::iterator lastIt=theMap.end(); //One past the end of the amp 00146 lastIt--;//end of map 00147 return fBinWidthInSeconds*(lastIt->first); 00148 00149 }
Generated on Tue Sep 11 19:51:09 2012 for ARA ROOT v3.3 Software by
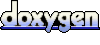